mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-05 15:27:42 +03:00
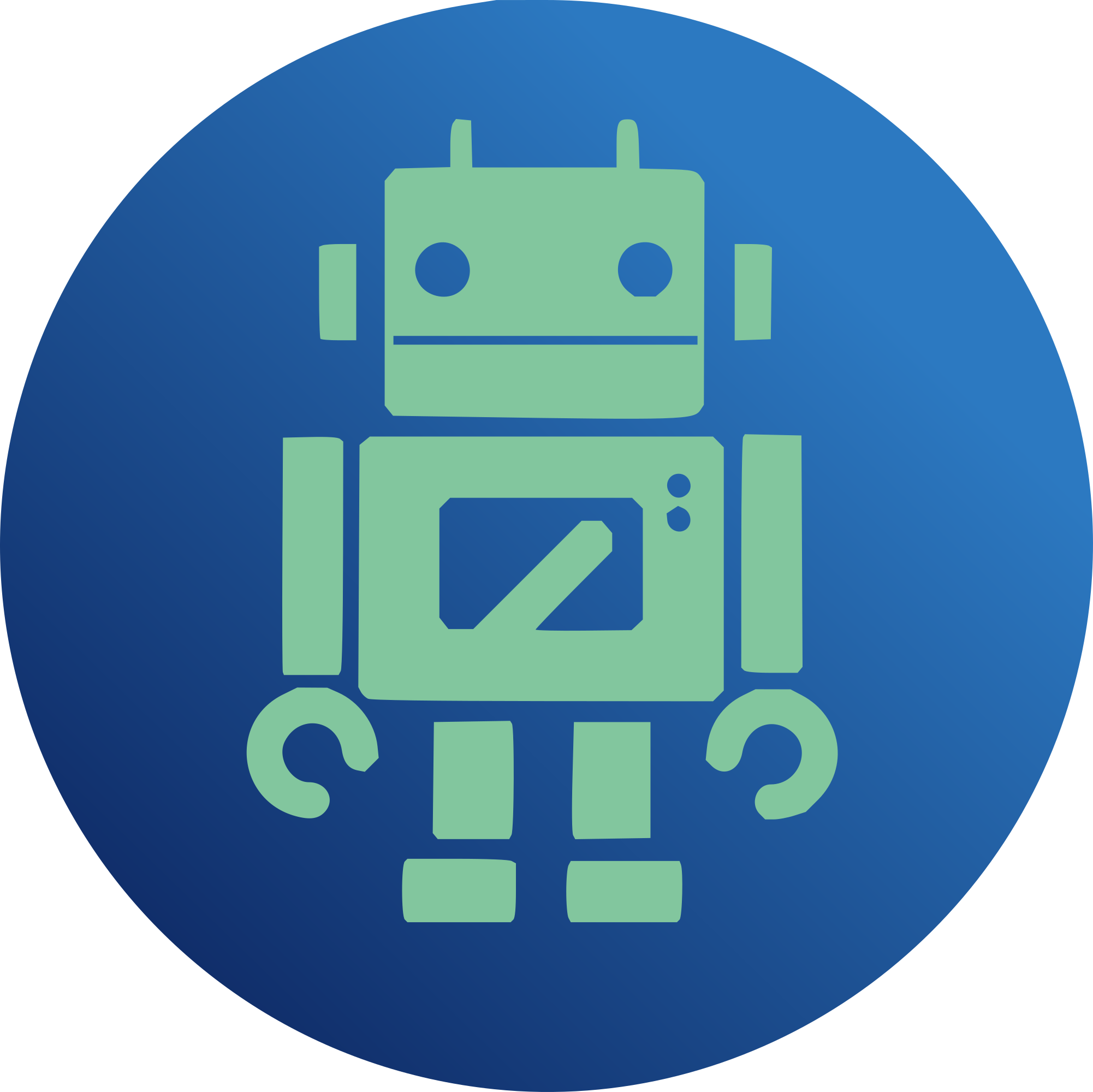
Previously Solaris style atomic primitives were implemented simply by wrapping the desired operation in a global spinlock. This was easy to implement at the time when I wasn't 100% sure I could safely layer the Solaris atomic primatives on the Linux counterparts. It however was likely not good for performance. After more investigation however it does appear the Solaris primitives can be layered on Linux's fairly safely. The Linux atomic_t type really just wraps a long so we can simply cast the Solaris unsigned value to either a atomic_t or atomic64_t. The only lingering problem for both implementations is that Solaris provides no atomic read function. This means reading a 64-bit value on a 32-bit arch can (and will) result in word breaking. I was very concerned about this initially, but upon further reflection it is a limitation of the Solaris API. So really we are just being bug-for-bug compatible here. With this change the default implementation is layered on top of Linux atomic types. However, because we're assuming a lot about the internal implementation of those types I've made it easy to fall-back to the generic approach. Simply build with --enable-atomic_spinlocks if issues are encountered with the new implementation.
43 lines
1.4 KiB
C
43 lines
1.4 KiB
C
/*
|
|
* This file is part of the SPL: Solaris Porting Layer.
|
|
*
|
|
* Copyright (c) 2008 Lawrence Livermore National Security, LLC.
|
|
* Produced at Lawrence Livermore National Laboratory
|
|
* Written by:
|
|
* Brian Behlendorf <behlendorf1@llnl.gov>,
|
|
* Herb Wartens <wartens2@llnl.gov>,
|
|
* Jim Garlick <garlick@llnl.gov>
|
|
* UCRL-CODE-235197
|
|
*
|
|
* This is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include <sys/atomic.h>
|
|
|
|
#ifdef DEBUG_SUBSYSTEM
|
|
#undef DEBUG_SUBSYSTEM
|
|
#endif
|
|
|
|
#define DEBUG_SUBSYSTEM S_ATOMIC
|
|
|
|
#ifdef ATOMIC_SPINLOCK
|
|
/* Global atomic lock declarations */
|
|
spinlock_t atomic32_lock = SPIN_LOCK_UNLOCKED;
|
|
spinlock_t atomic64_lock = SPIN_LOCK_UNLOCKED;
|
|
|
|
EXPORT_SYMBOL(atomic32_lock);
|
|
EXPORT_SYMBOL(atomic64_lock);
|
|
#endif /* ATOMIC_SPINLOCK */
|