mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-18 21:57:41 +03:00
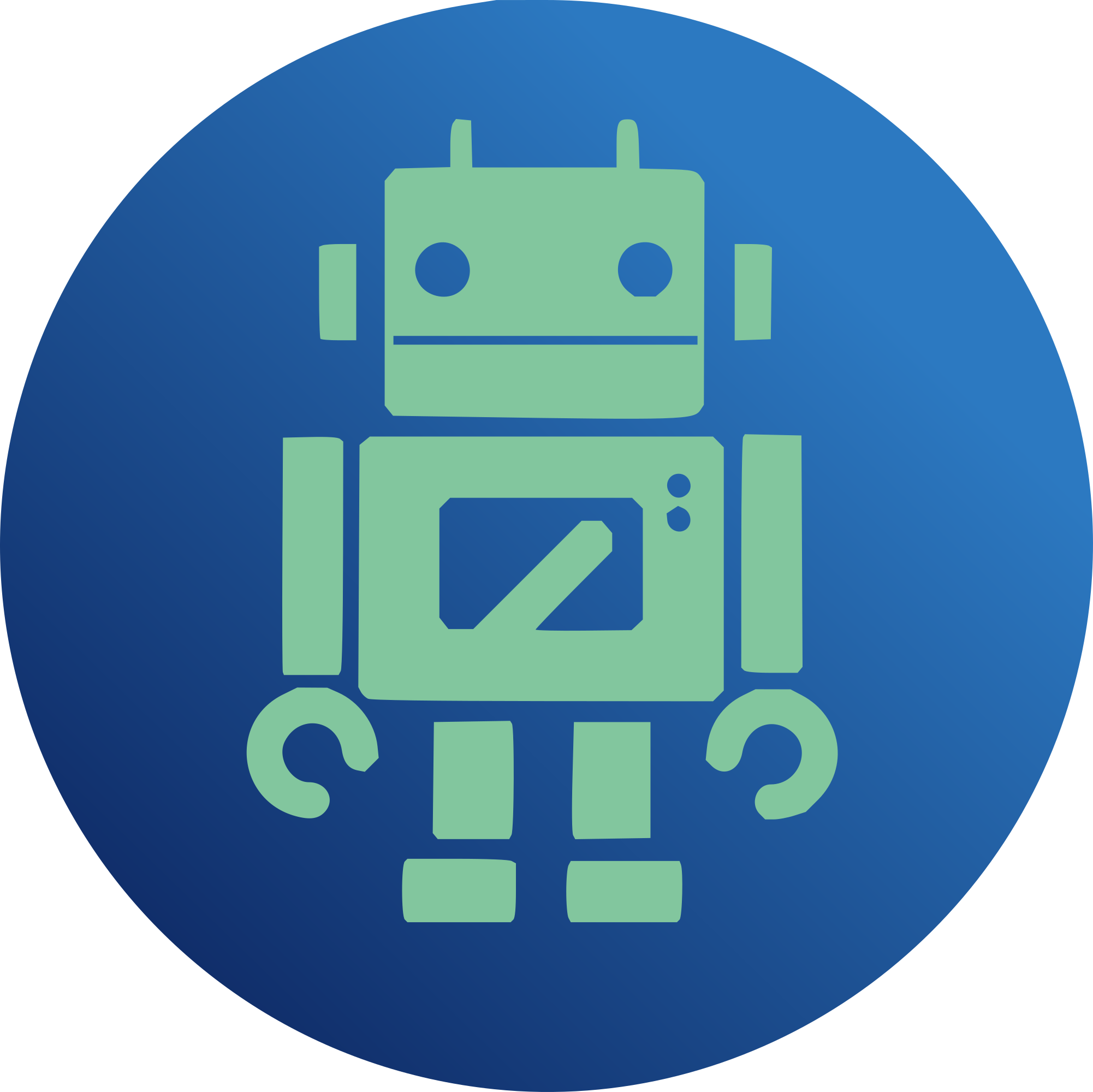
If multiple threads trip an assertion at the same moment (quite common), they can be printing at the same time, and their output gets messy. This adds a simple lock around the whole thing, to prevent a second task printing assert output before the first has finished. Additionally, if libspl_assert_ok is not set, abort() is called without dropping the lock, so that any other asserting tasks will be killed before starting any output, rather than only getting part-way through. This is a tradeoff; it's assumed that multiple threads asserting at the same moment are likely the same fault in different instances of a thread, and so there won't be any more useful information from the other tasks anyway. Reviewed-by: Brian Behlendorf <behlendorf1@llnl.gov> Signed-off-by: Rob Norris <robn@despairlabs.com> Sponsored-by: https://despairlabs.com/sponsor/ Closes #16140
94 lines
2.5 KiB
C
94 lines
2.5 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or https://opensource.org/licenses/CDDL-1.0.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright 2008 Sun Microsystems, Inc. All rights reserved.
|
|
* Use is subject to license terms.
|
|
*/
|
|
/*
|
|
* Copyright (c) 2024, Rob Norris <robn@despairlabs.com>
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <pthread.h>
|
|
|
|
#if defined(__linux__)
|
|
#include <errno.h>
|
|
#include <sys/prctl.h>
|
|
#ifdef HAVE_GETTID
|
|
#define libspl_gettid() gettid()
|
|
#else
|
|
#include <sys/syscall.h>
|
|
#define libspl_gettid() ((pid_t)syscall(__NR_gettid))
|
|
#endif
|
|
#define libspl_getprogname() (program_invocation_short_name)
|
|
#define libspl_getthreadname(buf, len) \
|
|
prctl(PR_GET_NAME, (unsigned long)(buf), 0, 0, 0)
|
|
#elif defined(__FreeBSD__)
|
|
#include <pthread_np.h>
|
|
#define libspl_gettid() pthread_getthreadid_np()
|
|
#define libspl_getprogname() getprogname()
|
|
#define libspl_getthreadname(buf, len) \
|
|
pthread_getname_np(pthread_self(), buf, len);
|
|
#endif
|
|
|
|
static boolean_t libspl_assert_ok = B_FALSE;
|
|
|
|
void
|
|
libspl_set_assert_ok(boolean_t val)
|
|
{
|
|
libspl_assert_ok = val;
|
|
}
|
|
|
|
static pthread_mutex_t assert_lock = PTHREAD_MUTEX_INITIALIZER;
|
|
|
|
/* printf version of libspl_assert */
|
|
void
|
|
libspl_assertf(const char *file, const char *func, int line,
|
|
const char *format, ...)
|
|
{
|
|
pthread_mutex_lock(&assert_lock);
|
|
|
|
va_list args;
|
|
char tname[64];
|
|
|
|
libspl_getthreadname(tname, sizeof (tname));
|
|
|
|
fprintf(stderr, "ASSERT at %s:%d:%s()\n", file, line, func);
|
|
|
|
va_start(args, format);
|
|
vfprintf(stderr, format, args);
|
|
va_end(args);
|
|
|
|
fprintf(stderr, "\n"
|
|
" PID: %-8u COMM: %s\n"
|
|
" TID: %-8u NAME: %s\n",
|
|
getpid(), libspl_getprogname(),
|
|
libspl_gettid(), tname);
|
|
|
|
#if !__has_feature(attribute_analyzer_noreturn) && !defined(__COVERITY__)
|
|
if (libspl_assert_ok) {
|
|
pthread_mutex_unlock(&assert_lock);
|
|
return;
|
|
}
|
|
#endif
|
|
abort();
|
|
}
|