mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 10:01:01 +03:00
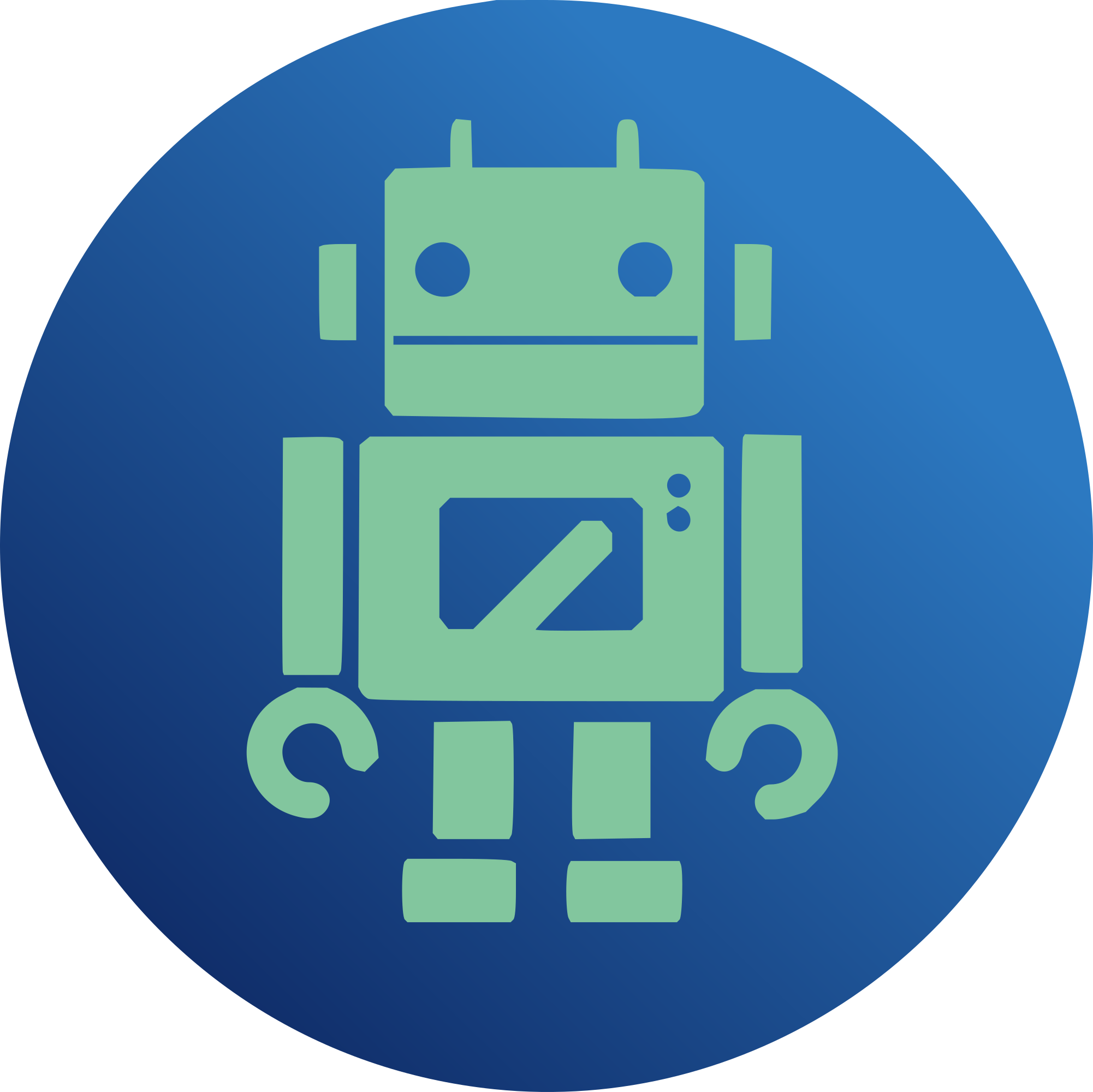
There exist a couple of macros that are used to update the blkptr birth times but they can often be confusing. For example, the BP_PHYSICAL_BIRTH() macro will provide either the physical birth time if it is set or else return back the logical birth time. The complement to this macro is BP_SET_BIRTH() which will set the logical birth time and set the physical birth time if they are not the same. Consumers may get confused when they are trying to get the physical birth time and use the BP_PHYSICAL_BIRTH() macro only to find out that the logical birth time is what is actually returned. This change cleans up these macros and makes them symmetrical. The same functionally is preserved but the name is changed. Instead of calling BP_PHYSICAL_BIRTH(), consumer can now call BP_GET_BIRTH(). In additional to cleaning up this naming conventions, two new sets of macros are introduced -- BP_[SET|GET]_LOGICAL_BIRTH() and BP_[SET|GET]_PHYSICAL_BIRTH. These new macros allow the consumer to get and set the specific birth time. As part of the cleanup, the unused GRID macros have been removed and that portion of the blkptr are currently unused. Reviewed-by: Matthew Ahrens <mahrens@delphix.com> Reviewed-by: Alexander Motin <mav@FreeBSD.org> Reviewed-by: Mark Maybee <mark.maybee@delphix.com> Signed-off-by: George Wilson <gwilson@delphix.com> Closes #15962
75 lines
2.2 KiB
C
75 lines
2.2 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or https://opensource.org/licenses/CDDL-1.0.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright (c) 2005, 2010, Oracle and/or its affiliates. All rights reserved.
|
|
* Copyright (c) 2013, 2017 by Delphix. All rights reserved.
|
|
*/
|
|
|
|
#include <sys/zfs_context.h>
|
|
#include <sys/uberblock_impl.h>
|
|
#include <sys/vdev_impl.h>
|
|
#include <sys/mmp.h>
|
|
|
|
int
|
|
uberblock_verify(uberblock_t *ub)
|
|
{
|
|
if (ub->ub_magic == BSWAP_64((uint64_t)UBERBLOCK_MAGIC))
|
|
byteswap_uint64_array(ub, sizeof (uberblock_t));
|
|
|
|
if (ub->ub_magic != UBERBLOCK_MAGIC)
|
|
return (SET_ERROR(EINVAL));
|
|
|
|
return (0);
|
|
}
|
|
|
|
/*
|
|
* Update the uberblock and return TRUE if anything changed in this
|
|
* transaction group.
|
|
*/
|
|
boolean_t
|
|
uberblock_update(uberblock_t *ub, vdev_t *rvd, uint64_t txg, uint64_t mmp_delay)
|
|
{
|
|
ASSERT(ub->ub_txg < txg);
|
|
|
|
/*
|
|
* We explicitly do not set ub_version here, so that older versions
|
|
* continue to be written with the previous uberblock version.
|
|
*/
|
|
ub->ub_magic = UBERBLOCK_MAGIC;
|
|
ub->ub_txg = txg;
|
|
ub->ub_guid_sum = rvd->vdev_guid_sum;
|
|
ub->ub_timestamp = gethrestime_sec();
|
|
ub->ub_software_version = SPA_VERSION;
|
|
ub->ub_mmp_magic = MMP_MAGIC;
|
|
if (spa_multihost(rvd->vdev_spa)) {
|
|
ub->ub_mmp_delay = mmp_delay;
|
|
ub->ub_mmp_config = MMP_SEQ_SET(0) |
|
|
MMP_INTERVAL_SET(zfs_multihost_interval) |
|
|
MMP_FAIL_INT_SET(zfs_multihost_fail_intervals);
|
|
} else {
|
|
ub->ub_mmp_delay = 0;
|
|
ub->ub_mmp_config = 0;
|
|
}
|
|
ub->ub_checkpoint_txg = 0;
|
|
|
|
return (BP_GET_LOGICAL_BIRTH(&ub->ub_rootbp) == txg);
|
|
}
|