mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-18 02:20:59 +03:00
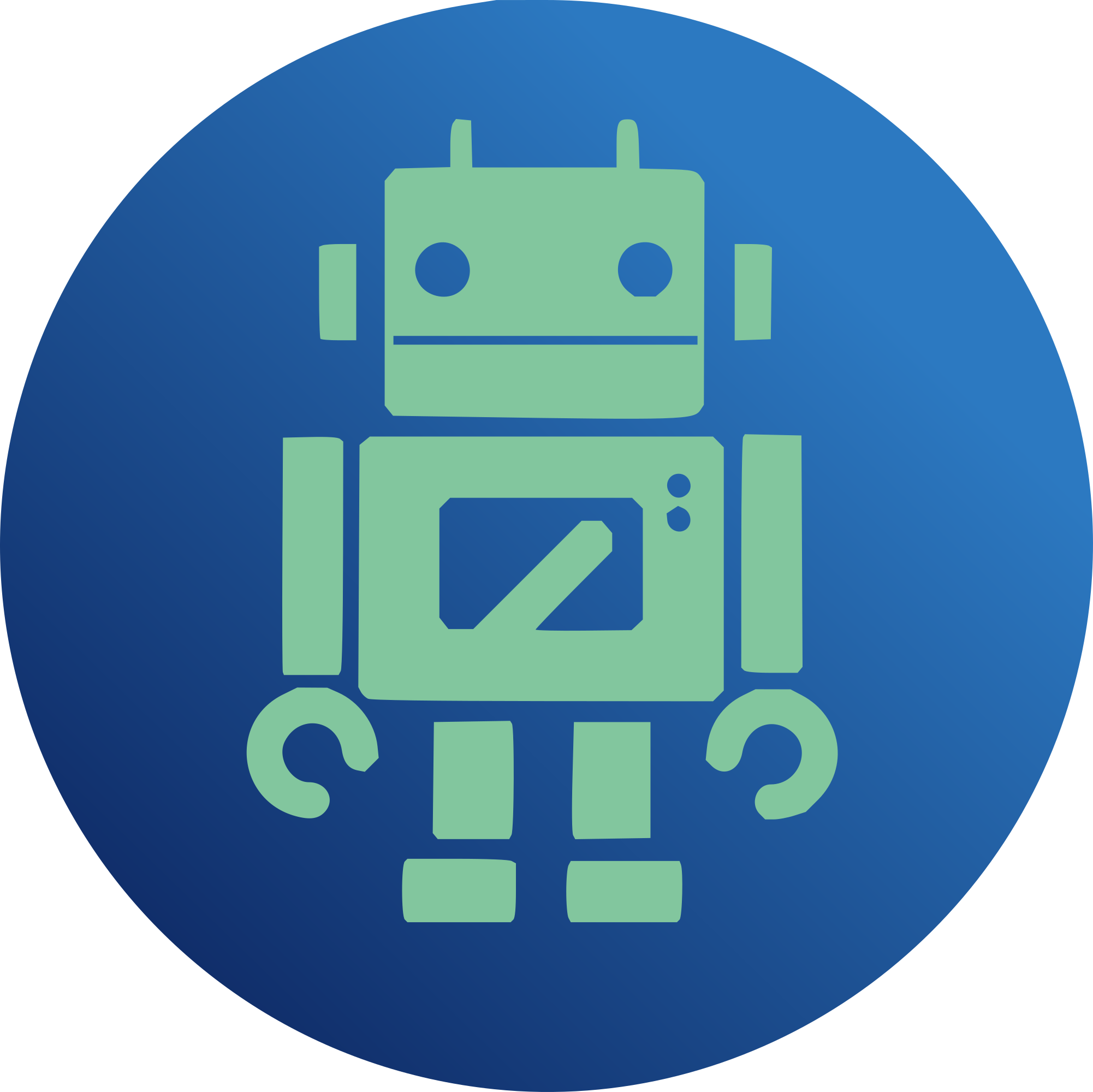
- In older systems without sysroot.mount, import before dracut-mount, and re-enable old dracut mount hook - rootflags MUST be present even if the administrator neglected to specify it explicitly - Check that mount.zfs exists in sbindir - Remove awk and head as (now unused) requirements, add grep, and install the right mount.zfs - Eliminate one use of grep in Dracut - Use a more accurate grepping statement to identify zfsutil in rootflags - Ensure that pooldev is nonempty - Properly handle /dev/sd* devices and more - Use new -P to get list of zpool devices - Bail out of the generator when zfs:AUTO is on the root command line - Ignore errors from systemctl trying to load sysroot.mount, we only care about the output - Determine which one is the correct initqueuedir at run time. - Add a compatibility getargbool for our detection / setup script. - Update dracut .gitignore files Signed-off-by: <Matthew Thode mthode@mthode.org> Signed-off-by: Brian Behlendorf <behlendorf1@llnl.gov> Closes #4558 Closes #4562
106 lines
2.2 KiB
Bash
Executable File
106 lines
2.2 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
command -v getarg >/dev/null || . /lib/dracut-lib.sh
|
|
command -v getargbool >/dev/null || {
|
|
# Compatibility with older Dracut versions.
|
|
# With apologies to the Dracut developers.
|
|
getargbool() {
|
|
local _b
|
|
unset _b
|
|
local _default
|
|
_default="$1"; shift
|
|
_b=$(getarg "$@")
|
|
[ $? -ne 0 -a -z "$_b" ] && _b="$_default"
|
|
if [ -n "$_b" ]; then
|
|
[ $_b = "0" ] && return 1
|
|
[ $_b = "no" ] && return 1
|
|
[ $_b = "off" ] && return 1
|
|
fi
|
|
return 0
|
|
}
|
|
}
|
|
|
|
OLDIFS="${IFS}"
|
|
NEWLINE="
|
|
"
|
|
|
|
ZPOOL_IMPORT_OPTS=""
|
|
if getargbool 0 zfs_force -y zfs.force -y zfsforce ; then
|
|
warn "ZFS: Will force-import pools if necessary."
|
|
ZPOOL_IMPORT_OPTS="${ZPOOL_IMPORT_OPTS} -f"
|
|
fi
|
|
|
|
# find_bootfs
|
|
# returns the first dataset with the bootfs attribute.
|
|
find_bootfs() {
|
|
IFS="${NEWLINE}"
|
|
for dataset in $(zpool list -H -o bootfs); do
|
|
case "${dataset}" in
|
|
"" | "-")
|
|
continue
|
|
;;
|
|
"no pools available")
|
|
IFS="${OLDIFS}"
|
|
return 1
|
|
;;
|
|
*)
|
|
IFS="${OLDIFS}"
|
|
echo "${dataset}"
|
|
return 0
|
|
;;
|
|
esac
|
|
done
|
|
|
|
IFS="${OLDIFS}"
|
|
return 1
|
|
}
|
|
|
|
# import_pool POOL
|
|
# imports the given zfs pool if it isn't imported already.
|
|
import_pool() {
|
|
local pool="${1}"
|
|
|
|
if ! zpool list -H "${pool}" 2>&1 > /dev/null ; then
|
|
info "ZFS: Importing pool ${pool}..."
|
|
if ! zpool import -N ${ZPOOL_IMPORT_OPTS} "${pool}" ; then
|
|
warn "ZFS: Unable to import pool ${pool}"
|
|
return 1
|
|
fi
|
|
fi
|
|
|
|
return 0
|
|
}
|
|
|
|
# mount_dataset DATASET
|
|
# mounts the given zfs dataset.
|
|
mount_dataset() {
|
|
local dataset="${1}"
|
|
local mountpoint="$(zfs get -H -o value mountpoint "${dataset}")"
|
|
|
|
# We need zfsutil for non-legacy mounts and not for legacy mounts.
|
|
if [ "${mountpoint}" = "legacy" ] ; then
|
|
mount -t zfs "${dataset}" "${NEWROOT}"
|
|
else
|
|
mount -o zfsutil -t zfs "${dataset}" "${NEWROOT}"
|
|
fi
|
|
|
|
return $?
|
|
}
|
|
|
|
# export_all OPTS
|
|
# exports all imported zfs pools.
|
|
export_all() {
|
|
local opts="${1}"
|
|
local ret=0
|
|
|
|
IFS="${NEWLINE}"
|
|
for pool in `zpool list -H -o name` ; do
|
|
if zpool list -H "${pool}" 2>&1 > /dev/null ; then
|
|
zpool export "${pool}" ${opts} || ret=$?
|
|
fi
|
|
done
|
|
IFS="${OLDIFS}"
|
|
|
|
return ${ret}
|
|
}
|