mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-18 10:21:01 +03:00
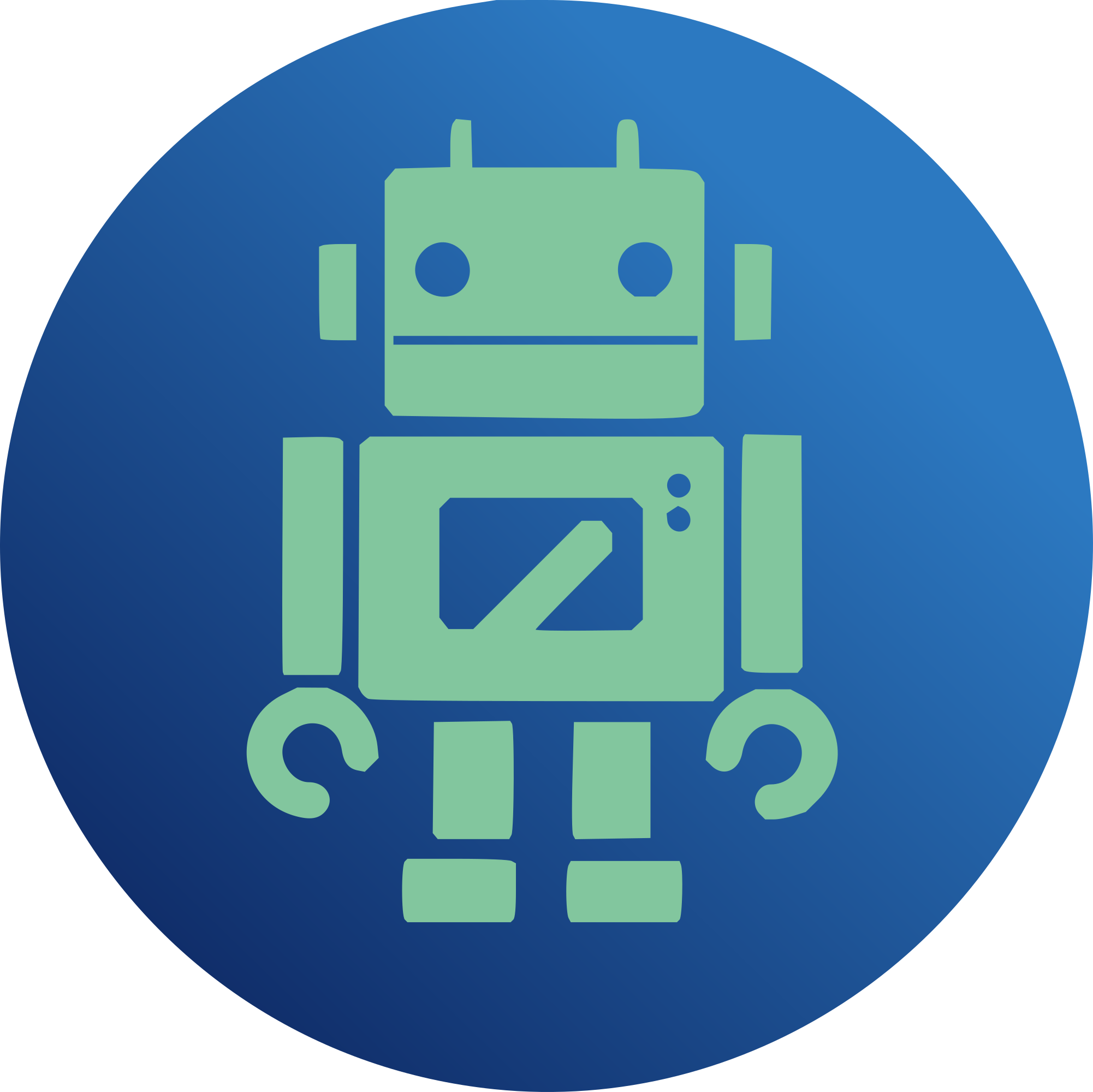
Contrary to initial testing we cannot rely on these kernels to invalidate the per-cpu FPU state and restore the FPU registers. Nor can we guarantee that the kernel won't modify the FPU state which we saved in the task struck. Therefore, the kfpu_begin() and kfpu_end() functions have been updated to save and restore the FPU state using our own dedicated per-cpu FPU state variables. This has the additional advantage of allowing us to use the FPU again in user threads. So we remove the code which was added to use task queues to ensure some functions ran in kernel threads. Reviewed-by: Fabian Grünbichler <f.gruenbichler@proxmox.com> Reviewed-by: Tony Hutter <hutter2@llnl.gov> Signed-off-by: Brian Behlendorf <behlendorf1@llnl.gov> Issue #9346 Closes #9403
76 lines
1.9 KiB
C
76 lines
1.9 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or http://www.opensolaris.org/os/licensing.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright (c) 2003, 2010, Oracle and/or its affiliates. All rights reserved.
|
|
*/
|
|
|
|
#ifndef _GCM_IMPL_H
|
|
#define _GCM_IMPL_H
|
|
|
|
/*
|
|
* GCM function dispatcher.
|
|
*/
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
#include <sys/zfs_context.h>
|
|
#include <sys/crypto/common.h>
|
|
|
|
/*
|
|
* Methods used to define GCM implementation
|
|
*
|
|
* @gcm_mul_f Perform carry-less multiplication
|
|
* @gcm_will_work_f Function tests whether implementation will function
|
|
*/
|
|
typedef void (*gcm_mul_f)(uint64_t *, uint64_t *, uint64_t *);
|
|
typedef boolean_t (*gcm_will_work_f)(void);
|
|
|
|
#define GCM_IMPL_NAME_MAX (16)
|
|
|
|
typedef struct gcm_impl_ops {
|
|
gcm_mul_f mul;
|
|
gcm_will_work_f is_supported;
|
|
char name[GCM_IMPL_NAME_MAX];
|
|
} gcm_impl_ops_t;
|
|
|
|
extern const gcm_impl_ops_t gcm_generic_impl;
|
|
#if defined(__x86_64) && defined(HAVE_PCLMULQDQ)
|
|
extern const gcm_impl_ops_t gcm_pclmulqdq_impl;
|
|
#endif
|
|
|
|
/*
|
|
* Initializes fastest implementation
|
|
*/
|
|
void gcm_impl_init(void);
|
|
|
|
/*
|
|
* Returns optimal allowed GCM implementation
|
|
*/
|
|
const struct gcm_impl_ops *gcm_impl_get_ops(void);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _GCM_IMPL_H */
|