mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 18:11:00 +03:00
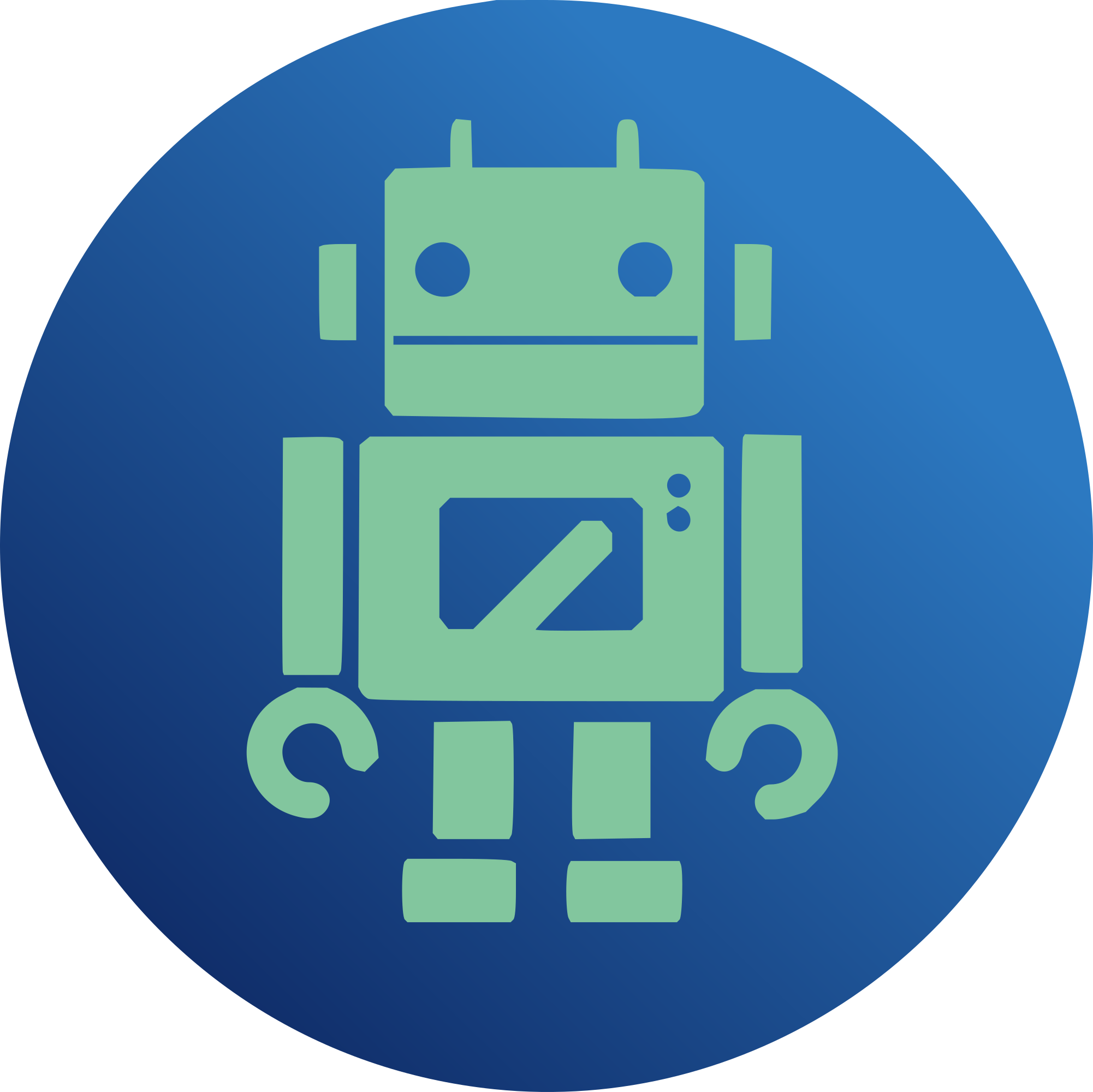
We need to use kthread_create() here for a few reasons. First off to old kernel_thread() API functioin will be going away. Secondly, and more importantly if I use kthread_create() we can then properly implement a thread_exit() function which terminates the kernel thread at any point with do_exit(). This fixes our cleanup bug which was caused by dropping a mutex twice after thread_exit() didn't really exit. git-svn-id: https://outreach.scidac.gov/svn/spl/trunk@66 7e1ea52c-4ff2-0310-8f11-9dd32ca42a1c
50 lines
1.2 KiB
C
50 lines
1.2 KiB
C
#ifndef _SPL_THREAD_H
|
|
#define _SPL_THREAD_H
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/kthread.h>
|
|
#include <sys/types.h>
|
|
#include <sys/sysmacros.h>
|
|
|
|
/*
|
|
* Thread interfaces
|
|
*/
|
|
#define TP_MAGIC 0x53535353
|
|
|
|
#define TS_SLEEP TASK_INTERRUPTIBLE
|
|
#define TS_RUN TASK_RUNNING
|
|
#define TS_ZOMB EXIT_ZOMBIE
|
|
#define TS_STOPPED TASK_STOPPED
|
|
#if 0
|
|
#define TS_FREE 0x00 /* No clean linux mapping */
|
|
#define TS_ONPROC 0x04 /* No clean linux mapping */
|
|
#define TS_WAIT 0x20 /* No clean linux mapping */
|
|
#endif
|
|
|
|
typedef void (*thread_func_t)(void *);
|
|
|
|
#define thread_create(stk, stksize, func, arg, len, pp, state, pri) \
|
|
__thread_create(stk, stksize, (thread_func_t)func, \
|
|
#func, arg, len, pp, state, pri)
|
|
#define thread_exit() __thread_exit()
|
|
#define curthread get_current()
|
|
|
|
extern kthread_t *__thread_create(caddr_t stk, size_t stksize,
|
|
thread_func_t func, const char *name,
|
|
void *args, size_t len, int *pp,
|
|
int state, pri_t pri);
|
|
extern void __thread_exit(void);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SPL_THREAD_H */
|
|
|