mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-01 21:47:36 +03:00
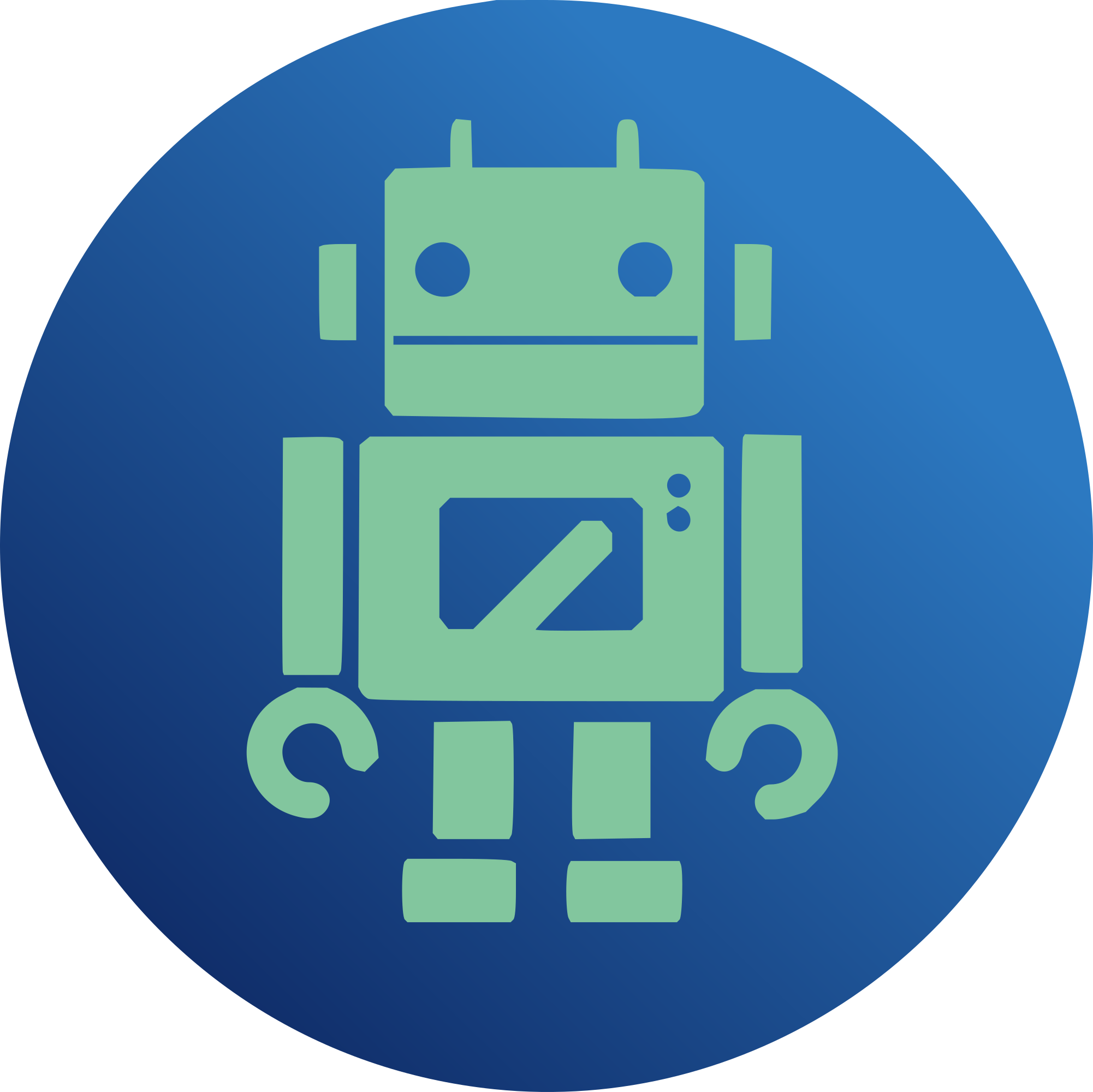
The ZFS range locking code in zfs_rlock.c/h depends on ZPL-specific data structures, specifically znode_t. However, it's also used by the ZVOL code, which uses a "dummy" znode_t to pass to the range locking code. We should clean this up so that the range locking code is generic and can be used equally by ZPL and ZVOL, and also can be used by future consumers that may need to run in userland (libzpool) as well as the kernel. Porting notes: * Added missing sys/avl.h include to sys/zfs_rlock.h. * Removed 'dbuf is within the locked range' ASSERTs from dmu_sync(). This was needed because ztest does not yet use a locked_range_t. * Removed "Approved by:" tag requirement from OpenZFS commit check to prevent needless warnings when integrating changes which has not been merged to illumos. * Reverted free_list range lock changes which were originally needed to defer the cv_destroy() which was called immediately after cv_broadcast(). Withd2733258
this should be safe but if not we may need to reintroduce this logic. * Reverts: The following two commits were reverted and squashed in to this change in order to make it easier to apply OpenZFS 9689. -d88895a0
, which removed the dummy znode from zvol_state -e3a07cd0
, which updated ztest to use range locks * Preserved optimized rangelock comparison function. Preserved the rangelock free list. The cv_destroy() function will block waiting for all processes in cv_wait() to be scheduled and drop their reference. This is done to ensure it's safe to free the condition variable. However, blocking while holding the rl->rl_lock mutex can result in a deadlock on Linux. A free list is introduced to defer the cv_destroy() and kmem_free() until after the mutex is released. Authored by: Matthew Ahrens <mahrens@delphix.com> Reviewed by: Brian Behlendorf <behlendorf1@llnl.gov> Reviewed by: Serapheim Dimitropoulos <serapheim.dimitro@delphix.com> Reviewed by: George Wilson <george.wilson@delphix.com> Reviewed by: Brad Lewis <brad.lewis@delphix.com> Ported-by: Brian Behlendorf <behlendorf1@llnl.gov> OpenZFS-issue: https://illumos.org/issues/9689 OpenZFS-commit: https://github.com/openzfs/openzfs/pull/680 External-issue: DLPX-58662 Closes #7980
219 lines
4.9 KiB
Bash
Executable File
219 lines
4.9 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
REF="HEAD"
|
|
|
|
# test a url
|
|
function test_url()
|
|
{
|
|
url="$1"
|
|
if ! curl --output /dev/null --max-time 60 \
|
|
--silent --head --fail "$url" ; then
|
|
echo "\"$url\" is unreachable"
|
|
return 1
|
|
fi
|
|
|
|
return 0
|
|
}
|
|
|
|
# test commit body for length
|
|
# lines containing urls are exempt for the length limit.
|
|
function test_commit_bodylength()
|
|
{
|
|
length="72"
|
|
body=$(git log -n 1 --pretty=%b "$REF" | grep -Ev "http(s)*://" | grep -E -m 1 ".{$((length + 1))}")
|
|
if [ -n "$body" ]; then
|
|
echo "error: commit message body contains line over ${length} characters"
|
|
return 1
|
|
fi
|
|
|
|
return 0
|
|
}
|
|
|
|
# check for a tagged line
|
|
function check_tagged_line()
|
|
{
|
|
regex='^\s*'"$1"':\s[[:print:]]+\s<[[:graph:]]+>$'
|
|
foundline=$(git log -n 1 "$REF" | grep -E -m 1 "$regex")
|
|
if [ -z "$foundline" ]; then
|
|
echo "error: missing \"$1\""
|
|
return 1
|
|
fi
|
|
|
|
return 0
|
|
}
|
|
|
|
# check for a tagged line and check that the link is valid
|
|
function check_tagged_line_with_url()
|
|
{
|
|
regex='^\s*'"$1"':\s\K([[:graph:]]+)$'
|
|
foundline=$(git log -n 1 "$REF" | grep -Po "$regex")
|
|
if [ -z "$foundline" ]; then
|
|
echo "error: missing \"$1\""
|
|
return 1
|
|
fi
|
|
|
|
OLDIFS=$IFS
|
|
IFS=$'\n'
|
|
for url in $(echo -e "$foundline"); do
|
|
if ! test_url "$url"; then
|
|
return 1
|
|
fi
|
|
done
|
|
IFS=$OLDIFS
|
|
|
|
return 0
|
|
}
|
|
|
|
# check commit message for a normal commit
|
|
function new_change_commit()
|
|
{
|
|
error=0
|
|
|
|
# subject is not longer than 50 characters
|
|
long_subject=$(git log -n 1 --pretty=%s "$REF" | grep -E -m 1 '.{51}')
|
|
if [ -n "$long_subject" ]; then
|
|
echo "error: commit subject over 50 characters"
|
|
error=1
|
|
fi
|
|
|
|
# need a signed off by
|
|
if ! check_tagged_line "Signed-off-by" ; then
|
|
error=1
|
|
fi
|
|
|
|
# ensure that no lines in the body of the commit are over 72 characters
|
|
if ! test_commit_bodylength ; then
|
|
error=1
|
|
fi
|
|
|
|
return $error
|
|
}
|
|
|
|
function is_openzfs_port()
|
|
{
|
|
# subject starts with OpenZFS means it's an openzfs port
|
|
subject=$(git log -n 1 --pretty=%s "$REF" | grep -E -m 1 '^OpenZFS')
|
|
if [ -n "$subject" ]; then
|
|
return 0
|
|
fi
|
|
|
|
return 1
|
|
}
|
|
|
|
function openzfs_port_commit()
|
|
{
|
|
error=0
|
|
|
|
# subject starts with OpenZFS dddd
|
|
subject=$(git log -n 1 --pretty=%s "$REF" | grep -E -m 1 '^OpenZFS [[:digit:]]+(, [[:digit:]]+)* - ')
|
|
if [ -z "$subject" ]; then
|
|
echo "error: OpenZFS patch ports must have a subject line that starts with \"OpenZFS dddd - \""
|
|
error=1
|
|
fi
|
|
|
|
# need an authored by line
|
|
if ! check_tagged_line "Authored by" ; then
|
|
error=1
|
|
fi
|
|
|
|
# need a reviewed by line
|
|
if ! check_tagged_line "Reviewed by" ; then
|
|
error=1
|
|
fi
|
|
|
|
# need ported by line
|
|
if ! check_tagged_line "Ported-by" ; then
|
|
error=1
|
|
fi
|
|
|
|
# need a url to openzfs commit and it should be valid
|
|
if ! check_tagged_line_with_url "OpenZFS-commit" ; then
|
|
error=1
|
|
fi
|
|
|
|
# need a url to illumos issue and it should be valid
|
|
if ! check_tagged_line_with_url "OpenZFS-issue" ; then
|
|
error=1
|
|
fi
|
|
|
|
return $error
|
|
}
|
|
|
|
function is_coverity_fix()
|
|
{
|
|
# subject starts with Fix coverity defects means it's a coverity fix
|
|
subject=$(git log -n 1 --pretty=%s "$REF" | grep -E -m 1 '^Fix coverity defects')
|
|
if [ -n "$subject" ]; then
|
|
return 0
|
|
fi
|
|
|
|
return 1
|
|
}
|
|
|
|
function coverity_fix_commit()
|
|
{
|
|
error=0
|
|
|
|
# subject starts with Fix coverity defects: CID dddd, dddd...
|
|
subject=$(git log -n 1 --pretty=%s "$REF" |
|
|
grep -E -m 1 'Fix coverity defects: CID [[:digit:]]+(, [[:digit:]]+)*')
|
|
if [ -z "$subject" ]; then
|
|
echo "error: Coverity defect fixes must have a subject line that starts with \"Fix coverity defects: CID dddd\""
|
|
error=1
|
|
fi
|
|
|
|
# need a signed off by
|
|
if ! check_tagged_line "Signed-off-by" ; then
|
|
error=1
|
|
fi
|
|
|
|
# test each summary line for the proper format
|
|
OLDIFS=$IFS
|
|
IFS=$'\n'
|
|
for line in $(git log -n 1 --pretty=%b "$REF" | grep -E '^CID'); do
|
|
echo "$line" | grep -E '^CID [[:digit:]]+: ([[:graph:]]+|[[:space:]])+ \(([[:upper:]]|\_)+\)' > /dev/null
|
|
# shellcheck disable=SC2181
|
|
if [[ $? -ne 0 ]]; then
|
|
echo "error: commit message has an improperly formatted CID defect line"
|
|
error=1
|
|
fi
|
|
done
|
|
IFS=$OLDIFS
|
|
|
|
# ensure that no lines in the body of the commit are over 72 characters
|
|
if ! test_commit_bodylength; then
|
|
error=1
|
|
fi
|
|
|
|
return $error
|
|
}
|
|
|
|
if [ -n "$1" ]; then
|
|
REF="$1"
|
|
fi
|
|
|
|
# if openzfs port, test against that
|
|
if is_openzfs_port; then
|
|
if ! openzfs_port_commit ; then
|
|
exit 1
|
|
else
|
|
exit 0
|
|
fi
|
|
fi
|
|
|
|
# if coverity fix, test against that
|
|
if is_coverity_fix; then
|
|
if ! coverity_fix_commit; then
|
|
exit 1
|
|
else
|
|
exit 0
|
|
fi
|
|
fi
|
|
|
|
# have a normal commit
|
|
if ! new_change_commit ; then
|
|
exit 1
|
|
fi
|
|
|
|
exit 0
|