mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-05 23:37:40 +03:00
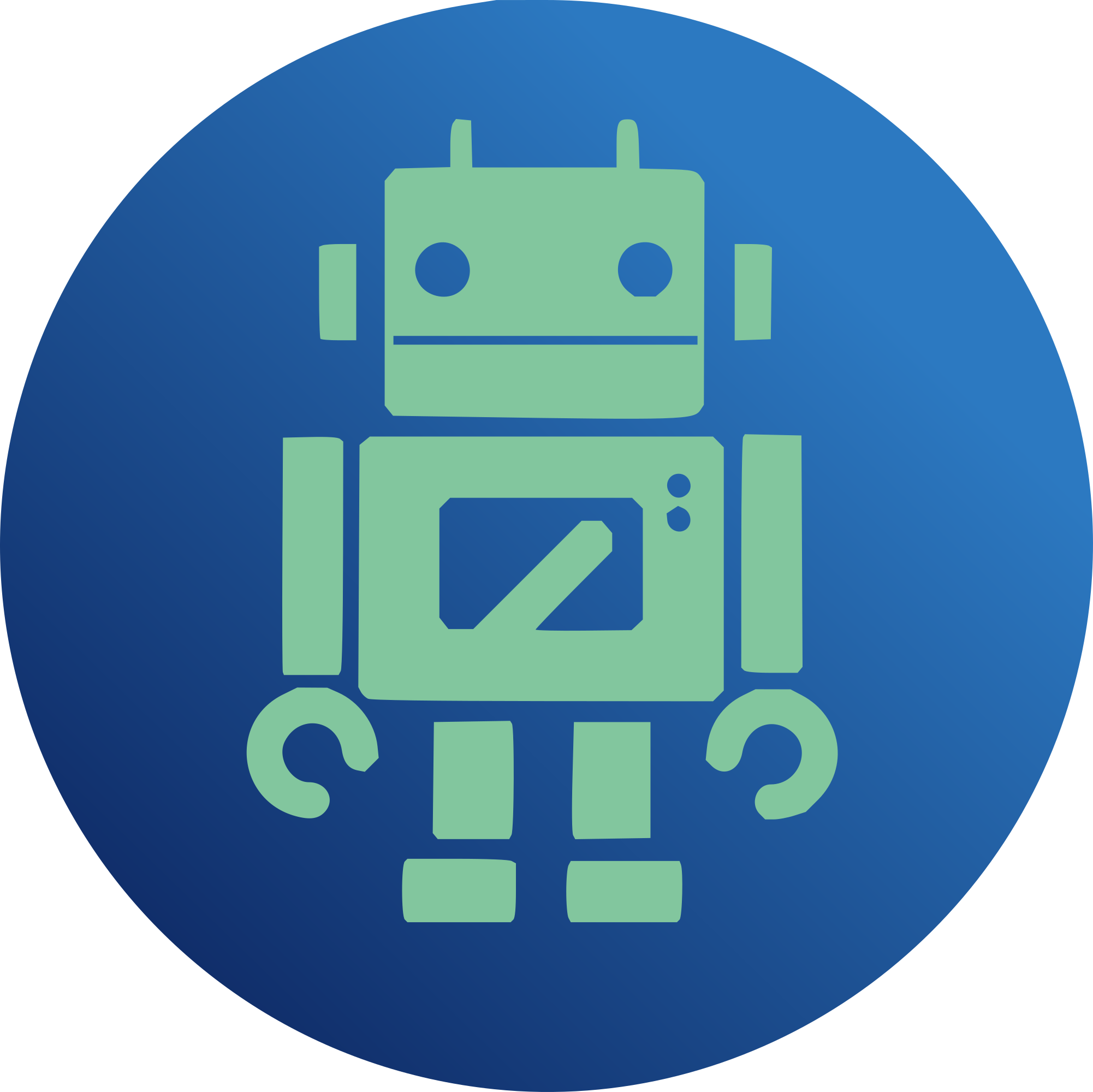
Update check.sh script to take V=1 env var so you can run it verbosely as follows if your chasing something: sudo make check V=1 Add new kobj api and needed regression tests to allow reading of files from within the kernel. Normally thats not something I support but the spa layer needs the support for its config file. Add some more missing stub headers git-svn-id: https://outreach.scidac.gov/svn/spl/trunk@38 7e1ea52c-4ff2-0310-8f11-9dd32ca42a1c
76 lines
1.5 KiB
C
76 lines
1.5 KiB
C
#include <sys/kobj.h>
|
|
#include "config.h"
|
|
|
|
void *rootdir = NULL;
|
|
EXPORT_SYMBOL(rootdir);
|
|
|
|
struct _buf *
|
|
kobj_open_file(const char *name)
|
|
{
|
|
struct _buf *file;
|
|
struct file *fp;
|
|
|
|
fp = filp_open(name, O_RDONLY, 0644);
|
|
if (IS_ERR(fp))
|
|
return ((_buf_t *)-1UL);
|
|
|
|
file = kmem_zalloc(sizeof(_buf_t), KM_SLEEP);
|
|
file->fp = fp;
|
|
|
|
return file;
|
|
} /* kobj_open_file() */
|
|
EXPORT_SYMBOL(kobj_open_file);
|
|
|
|
void
|
|
kobj_close_file(struct _buf *file)
|
|
{
|
|
filp_close(file->fp, 0);
|
|
kmem_free(file, sizeof(_buf_t));
|
|
|
|
return;
|
|
} /* kobj_close_file() */
|
|
EXPORT_SYMBOL(kobj_close_file);
|
|
|
|
int
|
|
kobj_read_file(struct _buf *file, char *buf, unsigned size, unsigned off)
|
|
{
|
|
loff_t offset = off;
|
|
mm_segment_t saved_fs;
|
|
int rc;
|
|
|
|
if (!file || !file->fp)
|
|
return -EINVAL;
|
|
|
|
if (!file->fp->f_op || !file->fp->f_op->read)
|
|
return -ENOSYS;
|
|
|
|
/* Writable user data segment must be briefly increased for this
|
|
* process so we can use the user space read call paths to write
|
|
* in to memory allocated by the kernel. */
|
|
saved_fs = get_fs();
|
|
set_fs(get_ds());
|
|
rc = file->fp->f_op->read(file->fp, buf, size, &offset);
|
|
set_fs(saved_fs);
|
|
|
|
return rc;
|
|
} /* kobj_read_file() */
|
|
EXPORT_SYMBOL(kobj_read_file);
|
|
|
|
int
|
|
kobj_get_filesize(struct _buf *file, uint64_t *size)
|
|
{
|
|
struct kstat stat;
|
|
int rc;
|
|
|
|
if (!file || !file->fp || !size)
|
|
return -EINVAL;
|
|
|
|
rc = vfs_getattr(file->fp->f_vfsmnt, file->fp->f_dentry, &stat);
|
|
if (rc)
|
|
return rc;
|
|
|
|
*size = stat.size;
|
|
return rc;
|
|
} /* kobj_get_filesize() */
|
|
EXPORT_SYMBOL(kobj_get_filesize);
|