mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 18:11:00 +03:00
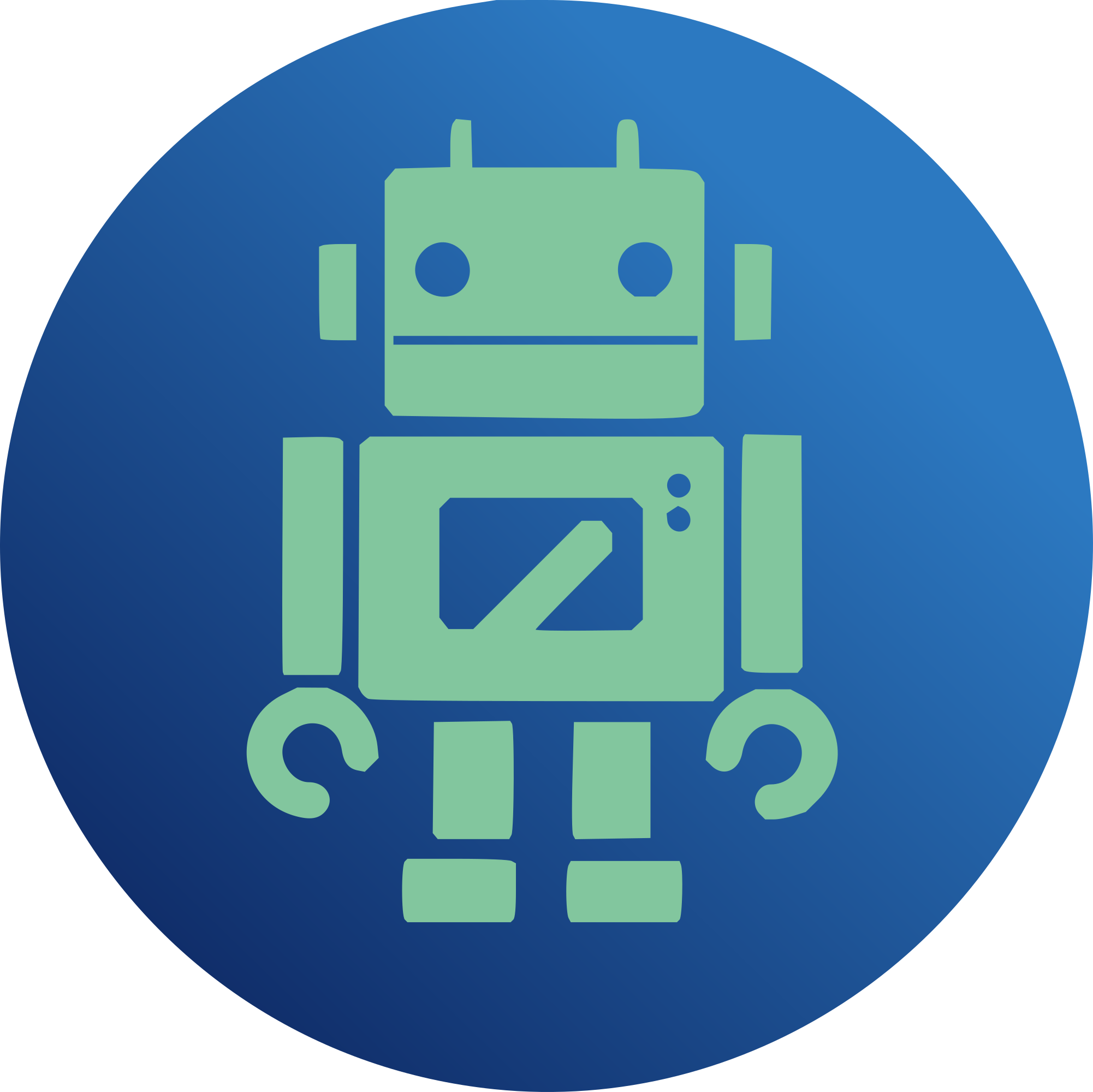
When Multihost is enabled, and a pool is imported, uberblock writes include ub_mmp_delay to allow an importing node to calculate the duration of an activity test. This value, is not enough information. If zfs_multihost_fail_intervals > 0 on the node with the pool imported, the safe minimum duration of the activity test is well defined, but does not depend on ub_mmp_delay: zfs_multihost_fail_intervals * zfs_multihost_interval and if zfs_multihost_fail_intervals == 0 on that node, there is no such well defined safe duration, but the importing host cannot tell whether mmp_delay is high due to I/O delays, or due to a very large zfs_multihost_interval setting on the host which last imported the pool. As a result, it may use a far longer period for the activity test than is necessary. This patch renames ub_mmp_sequence to ub_mmp_config and uses it to record the zfs_multihost_interval and zfs_multihost_fail_intervals values, as well as the mmp sequence. This allows a shorter activity test duration to be calculated by the importing host in most situations. These values are also added to the multihost_history kstat records. It calculates the activity test duration differently depending on whether the new fields are present or not; for importing pools with only ub_mmp_delay, it uses (zfs_multihost_interval + ub_mmp_delay) * zfs_multihost_import_intervals Which results in an activity test duration less sensitive to the leaf count. In addition, it makes a few other improvements: * It updates the "sequence" part of ub_mmp_config when MMP writes in between syncs occur. This allows an importing host to detect MMP on the remote host sooner, when the pool is idle, as it is not limited to the granularity of ub_timestamp (1 second). * It issues writes immediately when zfs_multihost_interval is changed so remote hosts see the updated value as soon as possible. * It fixes a bug where setting zfs_multihost_fail_intervals = 1 results in immediate pool suspension. * Update tests to verify activity check duration is based on recorded tunable values, not tunable values on importing host. * Update tests to verify the expected number of uberblocks have valid MMP fields - fail_intervals, mmp_interval, mmp_seq (sequence number), that sequence number is incrementing, and that uberblock values match tunable settings. Reviewed-by: Andreas Dilger <andreas.dilger@whamcloud.com> Reviewed-by: Brian Behlendorf <behlendorf1@llnl.gov> Reviewed-by: Tony Hutter <hutter2@llnl.gov> Signed-off-by: Olaf Faaland <faaland1@llnl.gov> Closes #7842
75 lines
2.3 KiB
C
75 lines
2.3 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* This file and its contents are supplied under the terms of the
|
|
* Common Development and Distribution License ("CDDL"), version 1.0.
|
|
* You may only use this file in accordance with the terms of version
|
|
* 1.0 of the CDDL.
|
|
*
|
|
* A full copy of the text of the CDDL should have accompanied this
|
|
* source. A copy of the CDDL is also available via the Internet at
|
|
* http://www.illumos.org/license/CDDL.
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright (C) 2017 by Lawrence Livermore National Security, LLC.
|
|
*/
|
|
|
|
#ifndef _SYS_MMP_H
|
|
#define _SYS_MMP_H
|
|
|
|
#include <sys/spa.h>
|
|
#include <sys/zfs_context.h>
|
|
#include <sys/uberblock_impl.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
#define MMP_MIN_INTERVAL 100 /* ms */
|
|
#define MMP_DEFAULT_INTERVAL 1000 /* ms */
|
|
#define MMP_DEFAULT_IMPORT_INTERVALS 20
|
|
#define MMP_DEFAULT_FAIL_INTERVALS 10
|
|
#define MMP_MIN_FAIL_INTERVALS 2 /* min if != 0 */
|
|
#define MMP_IMPORT_SAFETY_FACTOR 200 /* pct */
|
|
#define MMP_INTERVAL_OK(interval) MAX(interval, MMP_MIN_INTERVAL)
|
|
#define MMP_FAIL_INTVS_OK(fails) (fails == 0 ? 0 : MAX(fails, \
|
|
MMP_MIN_FAIL_INTERVALS))
|
|
|
|
typedef struct mmp_thread {
|
|
kmutex_t mmp_thread_lock; /* protect thread mgmt fields */
|
|
kcondvar_t mmp_thread_cv;
|
|
kthread_t *mmp_thread;
|
|
uint8_t mmp_thread_exiting;
|
|
kmutex_t mmp_io_lock; /* protect below */
|
|
hrtime_t mmp_last_write; /* last successful MMP write */
|
|
uint64_t mmp_delay; /* decaying avg ns between MMP writes */
|
|
uberblock_t mmp_ub; /* last ub written by sync */
|
|
zio_t *mmp_zio_root; /* root of mmp write zios */
|
|
uint64_t mmp_kstat_id; /* unique id for next MMP write kstat */
|
|
int mmp_skip_error; /* reason for last skipped write */
|
|
vdev_t *mmp_last_leaf; /* last mmp write sent here */
|
|
uint64_t mmp_leaf_last_gen; /* last mmp write sent here */
|
|
uint32_t mmp_seq; /* intra-second update counter */
|
|
} mmp_thread_t;
|
|
|
|
|
|
extern void mmp_init(struct spa *spa);
|
|
extern void mmp_fini(struct spa *spa);
|
|
extern void mmp_thread_start(struct spa *spa);
|
|
extern void mmp_thread_stop(struct spa *spa);
|
|
extern void mmp_update_uberblock(struct spa *spa, struct uberblock *ub);
|
|
extern void mmp_signal_all_threads(void);
|
|
|
|
/* Global tuning */
|
|
extern ulong_t zfs_multihost_interval;
|
|
extern uint_t zfs_multihost_fail_intervals;
|
|
extern uint_t zfs_multihost_import_intervals;
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SYS_MMP_H */
|