mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 10:01:01 +03:00
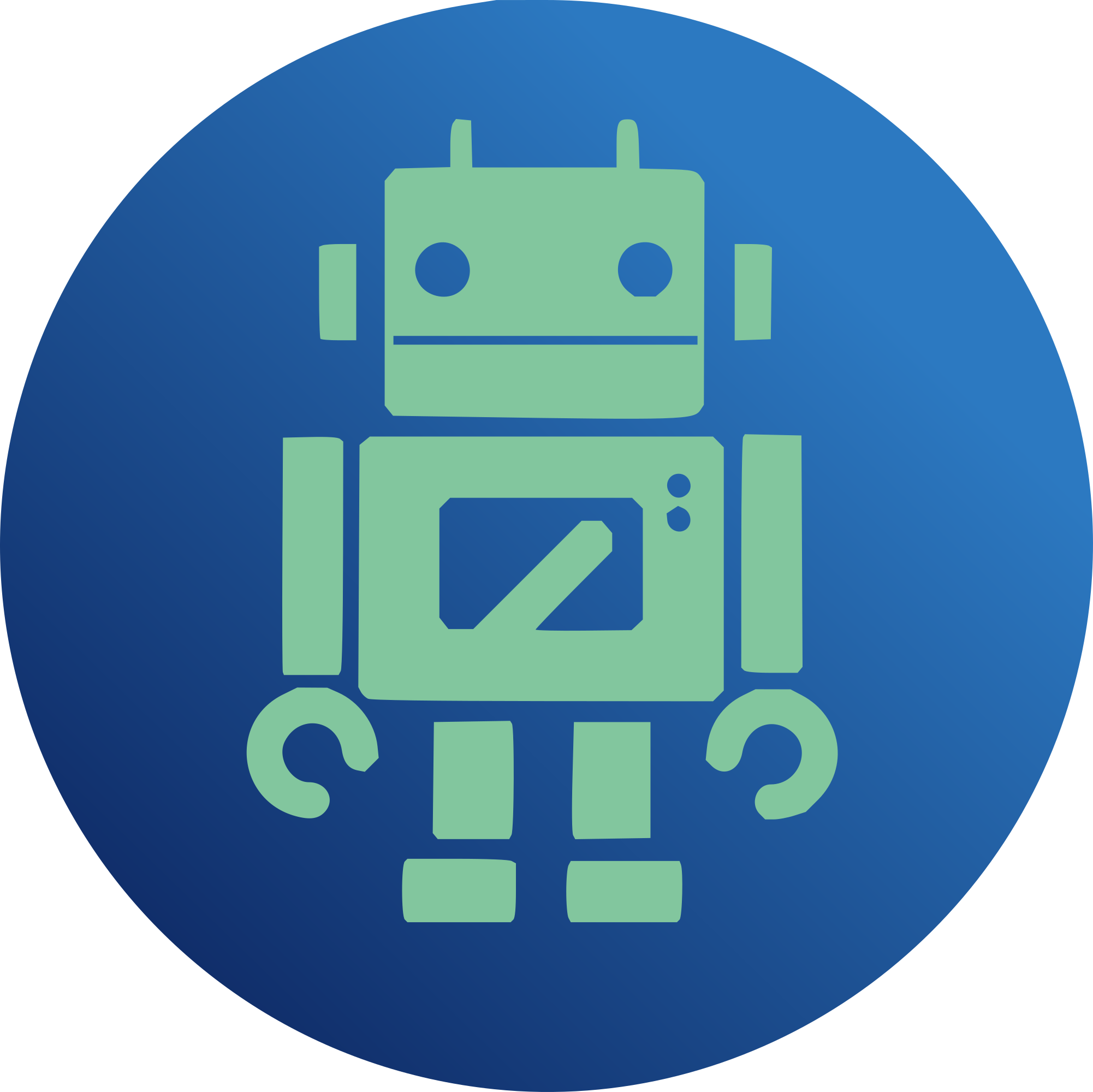
Some disks with internal sectors larger than 512 bytes (e.g., 4k) can suffer from bad write performance when ashift is not configured correctly. This is caused by the disk not reporting its actual sector size, but a sector size of 512 bytes. The drive may behave this way for compatibility reasons. For example, the WDC WD20EARS disks are known to exhibit this behavior. When creating a zpool, ZFS takes that wrong sector size and sets the "ashift" property accordingly (to 9: 1<<9=512), whereas it should be set to 12 for 4k sectors (1<<12=4096). This patch allows an adminstrator to manual specify the known correct ashift size at 'zpool create' time. This can significantly improve performance in certain cases. However, it will have an impact on your total pool capacity. See the updated ashift property description in the zpool.8 man page for additional details. Valid values for the ashift property range from 9 to 17 (512B-128KB). Additionally, you may set the ashift to 0 if you wish to auto-detect the sector size based on what the disk reports, this is the default behavior. The most common ashift values are 9 and 12. Example: zpool create -o ashift=12 tank raidz2 sda sdb sdc sdd Closes #280 Original-patch-by: Richard Laager <rlaager@wiktel.com> Signed-off-by: Brian Behlendorf <behlendorf1@llnl.gov>
73 lines
2.0 KiB
C
73 lines
2.0 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or http://www.opensolaris.org/os/licensing.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright (c) 2005, 2010, Oracle and/or its affiliates. All rights reserved.
|
|
*/
|
|
|
|
#ifndef ZPOOL_UTIL_H
|
|
#define ZPOOL_UTIL_H
|
|
|
|
#include <libnvpair.h>
|
|
#include <libzfs.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
/*
|
|
* Basic utility functions
|
|
*/
|
|
void *safe_malloc(size_t);
|
|
void zpool_no_memory(void);
|
|
uint_t num_logs(nvlist_t *nv);
|
|
|
|
/*
|
|
* Virtual device functions
|
|
*/
|
|
|
|
nvlist_t *make_root_vdev(zpool_handle_t *zhp, nvlist_t *props, int force,
|
|
int check_rep, boolean_t replacing, boolean_t dryrun, int argc, char **argv);
|
|
nvlist_t *split_mirror_vdev(zpool_handle_t *zhp, char *newname,
|
|
nvlist_t *props, splitflags_t flags, int argc, char **argv);
|
|
|
|
/*
|
|
* Pool list functions
|
|
*/
|
|
int for_each_pool(int, char **, boolean_t unavail, zprop_list_t **,
|
|
zpool_iter_f, void *);
|
|
|
|
typedef struct zpool_list zpool_list_t;
|
|
|
|
zpool_list_t *pool_list_get(int, char **, zprop_list_t **, int *);
|
|
void pool_list_update(zpool_list_t *);
|
|
int pool_list_iter(zpool_list_t *, int unavail, zpool_iter_f, void *);
|
|
void pool_list_free(zpool_list_t *);
|
|
int pool_list_count(zpool_list_t *);
|
|
void pool_list_remove(zpool_list_t *, zpool_handle_t *);
|
|
|
|
libzfs_handle_t *g_zfs;
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* ZPOOL_UTIL_H */
|