mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-19 02:41:00 +03:00
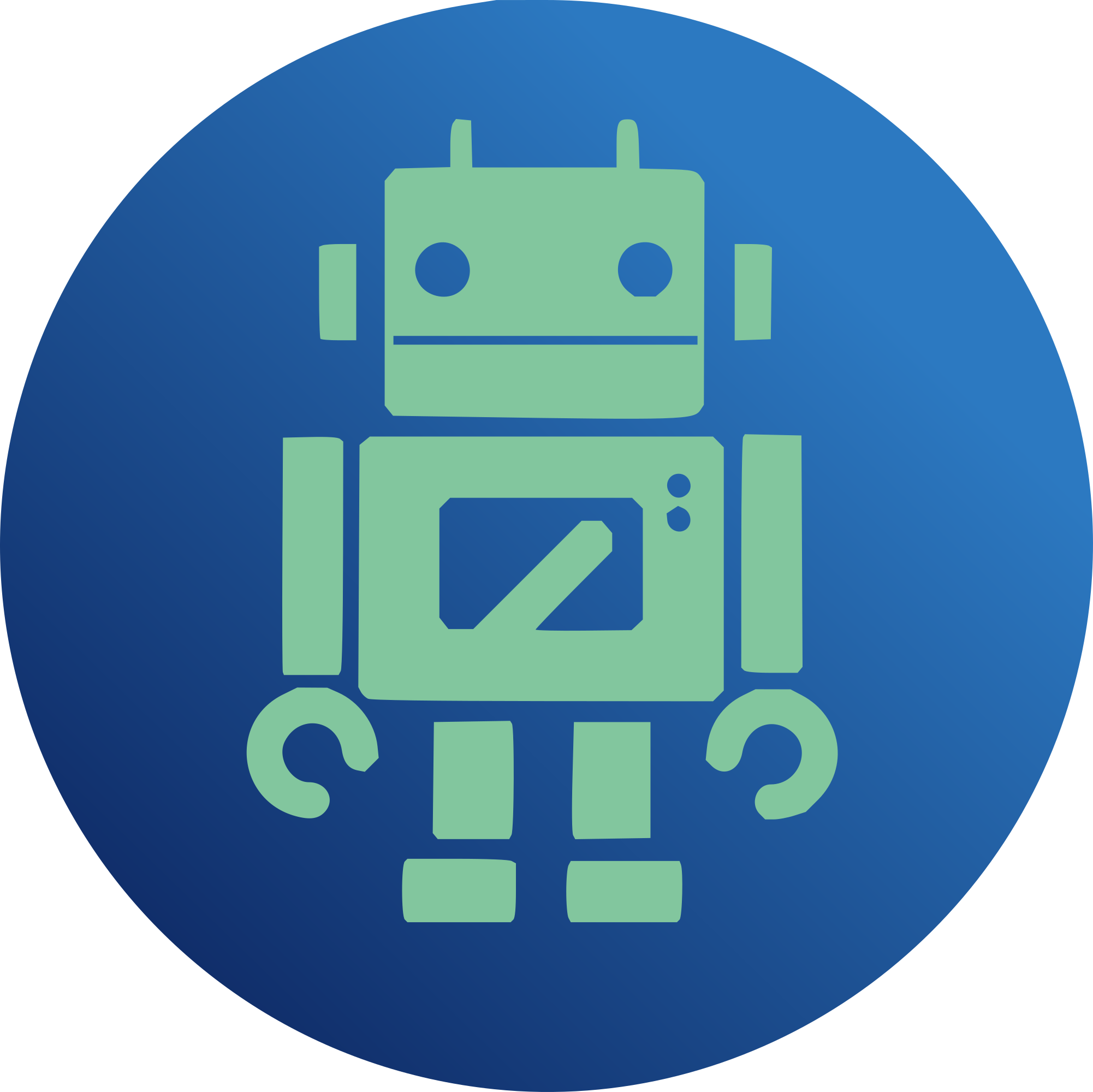
Update the common ZTS scripts and individual test cases as needed in order to allow them to be run on FreeBSD. The high level goal is to provide compatibility wrappers whenever possible to minimize changes to individual test cases. Reviewed-by: Brian Behlendorf <behlendorf1@llnl.gov> Reviewed-by: John Kennedy <john.kennedy@delphix.com> Signed-off-by: Matt Macy <mmacy@FreeBSD.org> Signed-off-by: Ryan Moeller <ryan@ixsystems.com> Closes #9692
67 lines
1.6 KiB
C
67 lines
1.6 KiB
C
/*
|
|
* This file and its contents are supplied under the terms of the
|
|
* Common Development and Distribution License ("CDDL"), version 1.0.
|
|
* You may only use this file in accordance with the terms of version
|
|
* 1.0 of the CDDL.
|
|
*
|
|
* A full copy of the text of the CDDL should have accompanied this
|
|
* source. A copy of the CDDL is also available via the Internet at
|
|
* http://www.illumos.org/license/CDDL.
|
|
*/
|
|
|
|
/*
|
|
* Copyright (c) 2016 by Delphix. All rights reserved.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <fcntl.h>
|
|
#include <string.h>
|
|
#include <errno.h>
|
|
#include <unistd.h>
|
|
#include <sys/param.h>
|
|
|
|
#define MAX_INT_LENGTH 10
|
|
|
|
static void
|
|
usage(char *msg, int exit_value)
|
|
{
|
|
(void) fprintf(stderr, "mkfiles basename max_file [min_file]\n");
|
|
(void) fprintf(stderr, "%s\n", msg);
|
|
exit(exit_value);
|
|
}
|
|
|
|
int
|
|
main(int argc, char **argv)
|
|
{
|
|
unsigned int numfiles = 0;
|
|
unsigned int first_file = 0;
|
|
unsigned int i;
|
|
char buf[MAXPATHLEN];
|
|
|
|
if (argc < 3 || argc > 4)
|
|
usage("Invalid number of arguments", -1);
|
|
|
|
if (sscanf(argv[2], "%u", &numfiles) != 1)
|
|
usage("Invalid maximum file", -2);
|
|
|
|
if (argc == 4 && sscanf(argv[3], "%u", &first_file) != 1)
|
|
usage("Invalid first file", -3);
|
|
|
|
for (i = first_file; i < first_file + numfiles; i++) {
|
|
int fd;
|
|
(void) snprintf(buf, MAXPATHLEN, "%s%u", argv[1], i);
|
|
if ((fd = open(buf, O_CREAT | O_EXCL, O_RDWR)) == -1) {
|
|
(void) fprintf(stderr, "Failed to create %s %s\n", buf,
|
|
strerror(errno));
|
|
return (-4);
|
|
} else if (fchown(fd, getuid(), getgid()) < 0) {
|
|
(void) fprintf(stderr, "Failed to chown %s %s\n", buf,
|
|
strerror(errno));
|
|
return (-5);
|
|
}
|
|
(void) close(fd);
|
|
}
|
|
return (0);
|
|
}
|