mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-15 20:27:40 +03:00
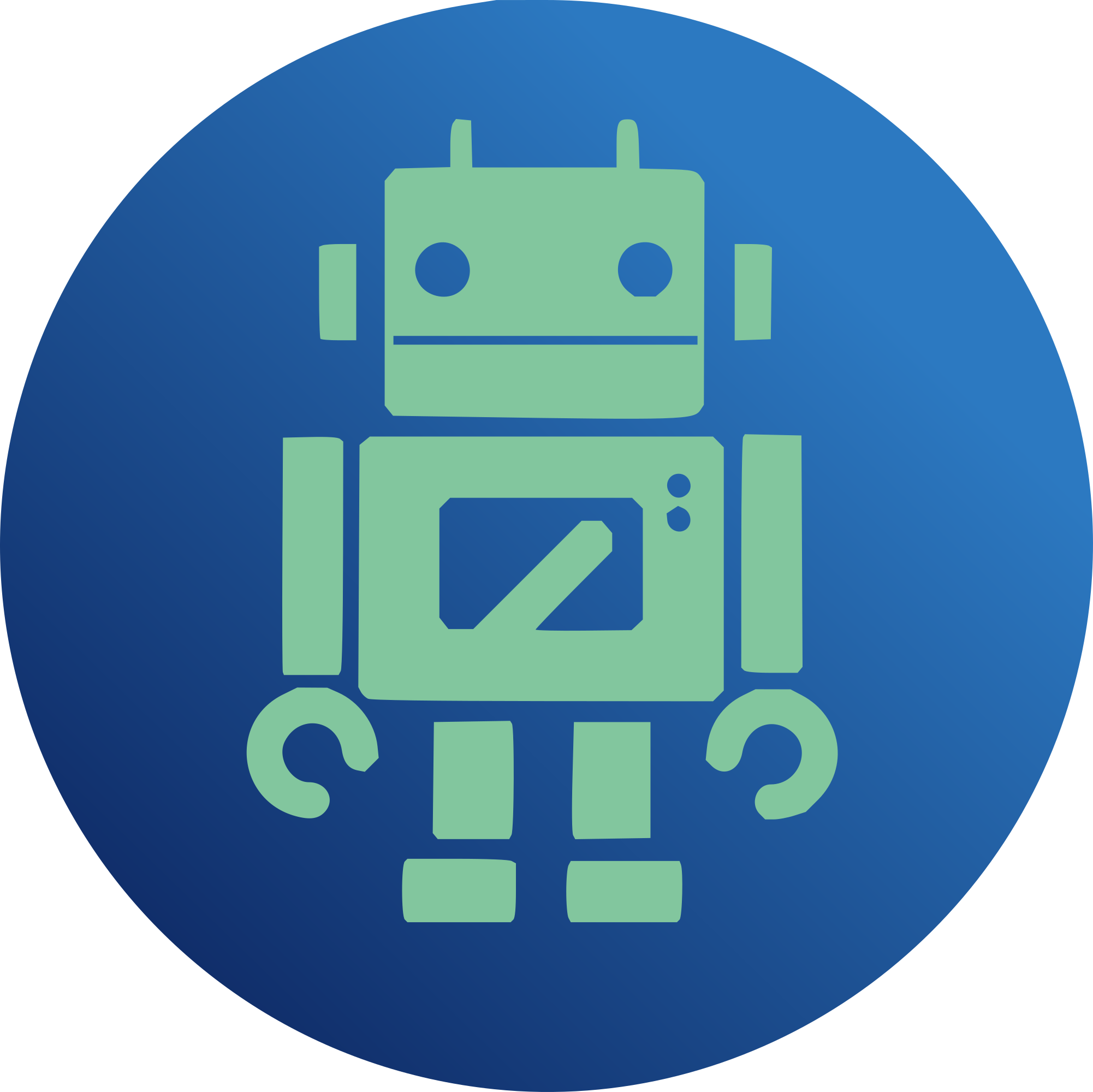
For a generic explanation of why mutexs needed to be reimplemented to work with the kernel lock profiling see commits:e811949a57
andd28db80fd0
The specific changes made to the mutex implemetation are as follows. The Linux mutex structure is now directly embedded in the kmutex_t. This allows a kmutex_t to be directly case to a mutex struct and passed directly to the Linux primative. Just like with the rwlocks it is critical that these functions be implemented as '#defines to ensure the location information is preserved. The preprocessor can then do a direct replacement of the Solaris primative with the linux primative. Just as with the rwlocks we need to track the lock owner. Here things get a little more interesting because depending on your kernel version, and how you've built your kernel Linux may already do this for you. If your running a 2.6.29 or newer kernel on a SMP system the lock owner will be tracked. This was added to Linux to support adaptive mutexs, more on that shortly. Alternately, your kernel might track the lock owner if you've set CONFIG_DEBUG_MUTEXES in the kernel build. If neither of the above things is true for your kernel the kmutex_t type will include and track the lock owner to ensure correct behavior. This is all handled by a new autoconf check called SPL_AC_MUTEX_OWNER. Concerning adaptive mutexs these are a very recent development and they did not make it in to either the latest FC11 of SLES11 kernels. Ideally, I'd love to see this kernel change appear in one of these distros because it does help performance. From Linux kernel commit: 0d66bf6d3514b35eb6897629059443132992dbd7 "Testing with Ingo's test-mutex application... gave a 345% boost for VFS scalability on my testbox" However, if you don't want to backport this change yourself you can still simply export the task_curr() symbol. The kmutex_t implementation will use this symbol when it's available to provide it's own adaptive mutexs. Finally, DEBUG_MUTEX support was removed including the proc handlers. This was done because now that we are cleanly integrated with the kernel profiling all this information and much much more is available in debug kernel builds. This code was now redundant. Update mutexs validated on: - SLES10 (ppc64) - SLES11 (x86_64) - CHAOS4.2 (x86_64) - RHEL5.3 (x86_64) - RHEL6 (x86_64) - FC11 (x86_64)
78 lines
2.8 KiB
C
78 lines
2.8 KiB
C
/*
|
|
* This file is part of the SPL: Solaris Porting Layer.
|
|
*
|
|
* Copyright (c) 2009 Lawrence Livermore National Security, LLC.
|
|
* Produced at Lawrence Livermore National Laboratory
|
|
* Written by:
|
|
* Brian Behlendorf <behlendorf1@llnl.gov>,
|
|
* Herb Wartens <wartens2@llnl.gov>,
|
|
* Jim Garlick <garlick@llnl.gov>
|
|
* UCRL-CODE-235197
|
|
*
|
|
* This is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include <sys/mutex.h>
|
|
|
|
#ifdef DEBUG_SUBSYSTEM
|
|
#undef DEBUG_SUBSYSTEM
|
|
#endif
|
|
|
|
#define DEBUG_SUBSYSTEM S_MUTEX
|
|
|
|
/*
|
|
* While a standard mutex implementation has been available in the kernel
|
|
* for quite some time. It was not until 2.6.29 and latter kernels that
|
|
* adaptive mutexs were embraced and integrated with the scheduler. This
|
|
* brought a significant performance improvement, but just as importantly
|
|
* it added a lock owner to the generic mutex outside CONFIG_DEBUG_MUTEXES
|
|
* builds. This is critical for correctly supporting the mutex_owner()
|
|
* Solaris primitive. When the owner is available we use a pure Linux
|
|
* mutex implementation. When the owner is not available we still use
|
|
* Linux mutexs as a base but also reserve space for an owner field right
|
|
* after the mutex structure.
|
|
*
|
|
* In the case when HAVE_MUTEX_OWNER is not defined your code may
|
|
* still me able to leverage adaptive mutexs. As long as the task_curr()
|
|
* symbol is exported this code will provide a poor mans adaptive mutex
|
|
* implementation. However, this is not required and if the symbol is
|
|
* unavailable we provide a standard mutex.
|
|
*/
|
|
|
|
#ifndef HAVE_MUTEX_OWNER
|
|
#ifdef HAVE_TASK_CURR
|
|
/*
|
|
* mutex_spin_max = { 0, -1, 1-MAX_INT }
|
|
* 0: Never spin when trying to acquire lock
|
|
* -1: Spin until acquired or holder yields without dropping lock
|
|
* 1-MAX_INT: Spin for N attempts before sleeping for lock
|
|
*/
|
|
int mutex_spin_max = 0;
|
|
module_param(mutex_spin_max, int, 0644);
|
|
MODULE_PARM_DESC(mutex_spin_max, "Spin a maximum of N times to acquire lock");
|
|
|
|
int
|
|
spl_mutex_spin_max(void)
|
|
{
|
|
return mutex_spin_max;
|
|
}
|
|
EXPORT_SYMBOL(spl_mutex_spin_max);
|
|
|
|
#endif /* HAVE_TASK_CURR */
|
|
#endif /* !HAVE_MUTEX_OWNER */
|
|
|
|
int spl_mutex_init(void) { return 0; }
|
|
void spl_mutex_fini(void) { }
|