mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2025-07-09 09:17:40 +03:00
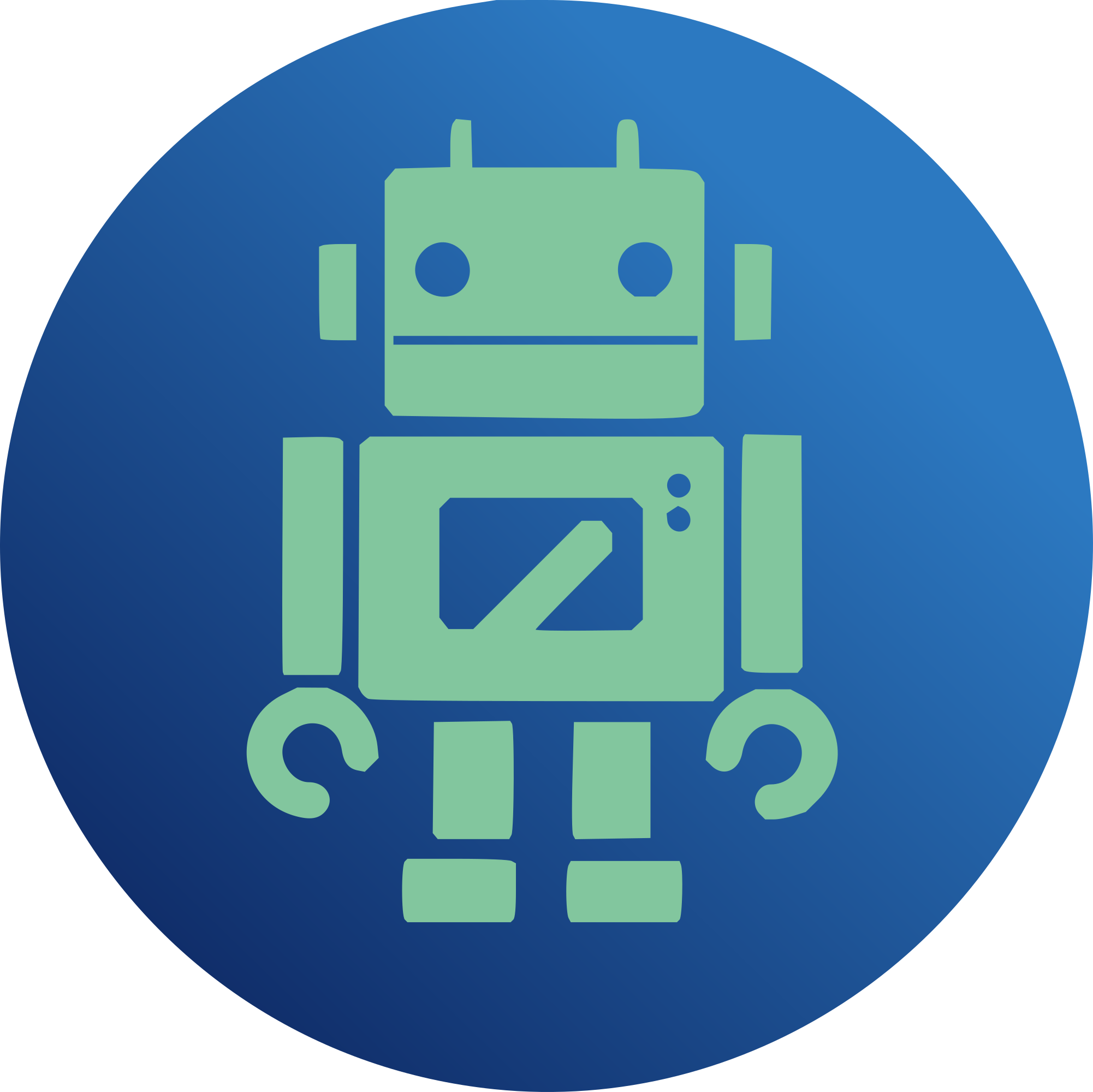
well for the expected workloads. Improvement in this commit include: - Added DEBUG_KMEM_TRACKING #define which can optionally be set when DEBUG_KMEM is defined to do per allocation tracking. This allows us to get all the lightweight kmem debugging enabled by default which is pretty light weight, and only when looking for a memory leak we can briefly enable the per alloc tracking. - Added set_normalized_timespec() in to SPL to simply using the timespec() primatives from within a module. - Added per-spinlock cycle counters to the slab in an attempt to run down a lock contention issue. The contended lock was in vmalloc() but I'm going to leave the cycle counters in place for a little while until I'm convinced there arn't other locking improvement possible in the slab. - Added a proc interface to the slab to export per slab cache statistics to /proc/spl/kmem/slab for analysis. - Reworked spl_slab_alloc() function to allocate from kmem for small allocation and vmem for large allocations. This improved things considerably but futher work is needed. git-svn-id: https://outreach.scidac.gov/svn/spl/trunk@138 7e1ea52c-4ff2-0310-8f11-9dd32ca42a1c
88 lines
2.5 KiB
C
88 lines
2.5 KiB
C
/*
|
|
* This file is part of the SPL: Solaris Porting Layer.
|
|
*
|
|
* Copyright (c) 2008 Lawrence Livermore National Security, LLC.
|
|
* Produced at Lawrence Livermore National Laboratory
|
|
* Written by:
|
|
* Brian Behlendorf <behlendorf1@llnl.gov>,
|
|
* Herb Wartens <wartens2@llnl.gov>,
|
|
* Jim Garlick <garlick@llnl.gov>
|
|
* UCRL-CODE-235197
|
|
*
|
|
* This is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include <sys/sysmacros.h>
|
|
#include <sys/time.h>
|
|
|
|
#ifdef DEBUG_SUBSYSTEM
|
|
#undef DEBUG_SUBSYSTEM
|
|
#endif
|
|
|
|
#define DEBUG_SUBSYSTEM S_TIME
|
|
|
|
void
|
|
__gethrestime(timestruc_t *ts)
|
|
{
|
|
getnstimeofday((struct timespec *)ts);
|
|
}
|
|
EXPORT_SYMBOL(__gethrestime);
|
|
|
|
int
|
|
__clock_gettime(clock_type_t type, timespec_t *tp)
|
|
{
|
|
/* Only support CLOCK_REALTIME+__CLOCK_REALTIME0 for now */
|
|
ASSERT((type == CLOCK_REALTIME) || (type == __CLOCK_REALTIME0));
|
|
|
|
getnstimeofday(tp);
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(__clock_gettime);
|
|
|
|
/* This function may not be as fast as using monotonic_clock() but it
|
|
* should be much more portable, if performance becomes as issue we can
|
|
* look at using monotonic_clock() for x86_64 and x86 arches.
|
|
*/
|
|
hrtime_t
|
|
__gethrtime(void) {
|
|
timespec_t tv;
|
|
hrtime_t rc;
|
|
|
|
do_posix_clock_monotonic_gettime(&tv);
|
|
rc = (NSEC_PER_SEC * (hrtime_t)tv.tv_sec) + (hrtime_t)tv.tv_nsec;
|
|
|
|
return rc;
|
|
}
|
|
EXPORT_SYMBOL(__gethrtime);
|
|
|
|
/* Not exported from the kernel, but we need it for timespec_sub. Be very
|
|
* careful here we are using the kernel prototype, so that must not change.
|
|
*/
|
|
void
|
|
set_normalized_timespec(struct timespec *ts, time_t sec, long nsec)
|
|
{
|
|
while (nsec >= NSEC_PER_SEC) {
|
|
nsec -= NSEC_PER_SEC;
|
|
++sec;
|
|
}
|
|
while (nsec < 0) {
|
|
nsec += NSEC_PER_SEC;
|
|
--sec;
|
|
}
|
|
ts->tv_sec = sec;
|
|
ts->tv_nsec = nsec;
|
|
}
|
|
EXPORT_SYMBOL(set_normalized_timespec);
|