mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 01:51:00 +03:00
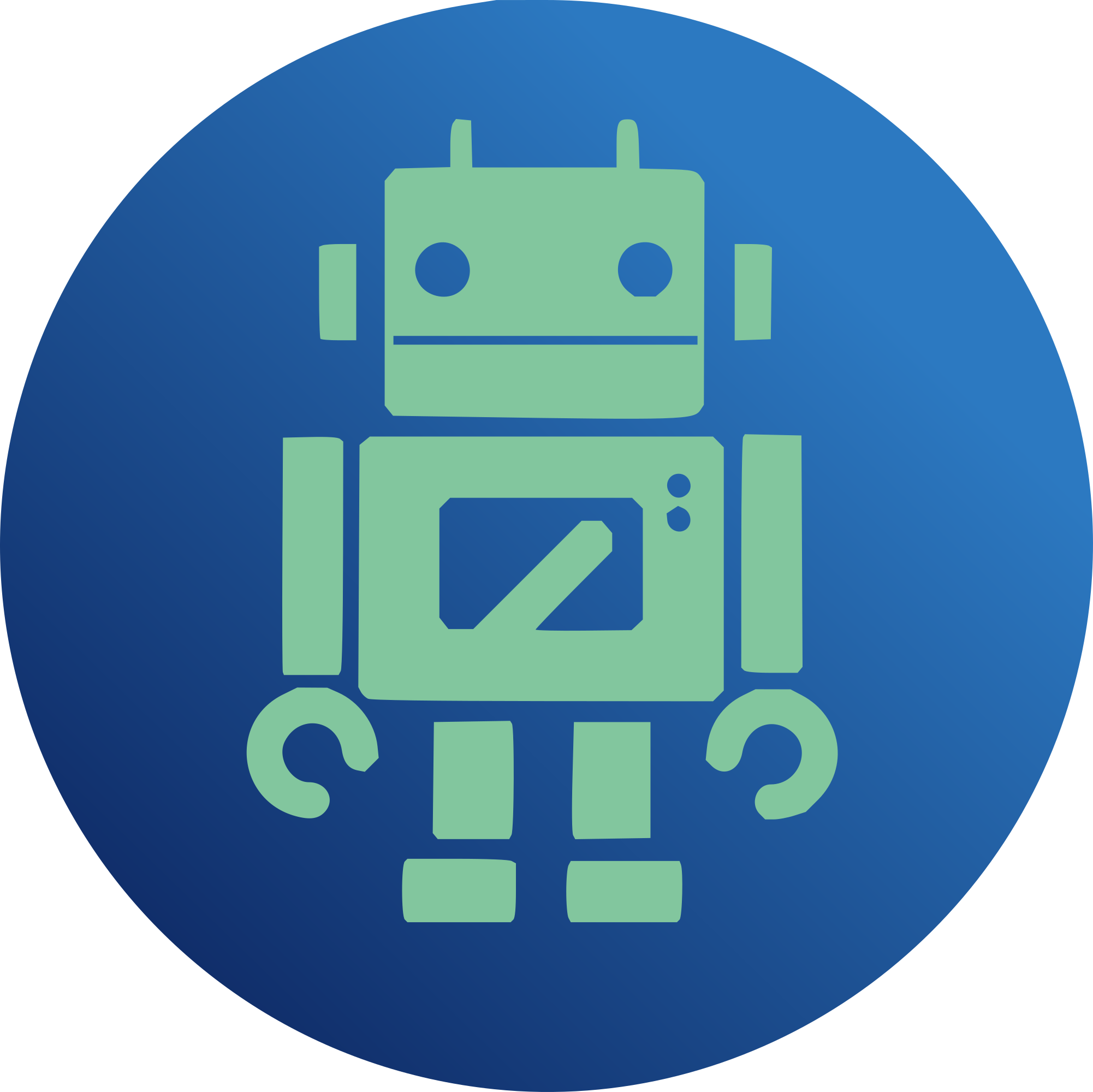
This feature allows disks to be added one at a time to a RAID-Z group, expanding its capacity incrementally. This feature is especially useful for small pools (typically with only one RAID-Z group), where there isn't sufficient hardware to add capacity by adding a whole new RAID-Z group (typically doubling the number of disks). == Initiating expansion == A new device (disk) can be attached to an existing RAIDZ vdev, by running `zpool attach POOL raidzP-N NEW_DEVICE`, e.g. `zpool attach tank raidz2-0 sda`. The new device will become part of the RAIDZ group. A "raidz expansion" will be initiated, and the new device will contribute additional space to the RAIDZ group once the expansion completes. The `feature@raidz_expansion` on-disk feature flag must be `enabled` to initiate an expansion, and it remains `active` for the life of the pool. In other words, pools with expanded RAIDZ vdevs can not be imported by older releases of the ZFS software. == During expansion == The expansion entails reading all allocated space from existing disks in the RAIDZ group, and rewriting it to the new disks in the RAIDZ group (including the newly added device). The expansion progress can be monitored with `zpool status`. Data redundancy is maintained during (and after) the expansion. If a disk fails while the expansion is in progress, the expansion pauses until the health of the RAIDZ vdev is restored (e.g. by replacing the failed disk and waiting for reconstruction to complete). The pool remains accessible during expansion. Following a reboot or export/import, the expansion resumes where it left off. == After expansion == When the expansion completes, the additional space is available for use, and is reflected in the `available` zfs property (as seen in `zfs list`, `df`, etc). Expansion does not change the number of failures that can be tolerated without data loss (e.g. a RAIDZ2 is still a RAIDZ2 even after expansion). A RAIDZ vdev can be expanded multiple times. After the expansion completes, old blocks remain with their old data-to-parity ratio (e.g. 5-wide RAIDZ2, has 3 data to 2 parity), but distributed among the larger set of disks. New blocks will be written with the new data-to-parity ratio (e.g. a 5-wide RAIDZ2 which has been expanded once to 6-wide, has 4 data to 2 parity). However, the RAIDZ vdev's "assumed parity ratio" does not change, so slightly less space than is expected may be reported for newly-written blocks, according to `zfs list`, `df`, `ls -s`, and similar tools. Sponsored-by: The FreeBSD Foundation Sponsored-by: iXsystems, Inc. Sponsored-by: vStack Reviewed-by: Brian Behlendorf <behlendorf1@llnl.gov> Reviewed-by: Mark Maybee <mark.maybee@delphix.com> Authored-by: Matthew Ahrens <mahrens@delphix.com> Contributions-by: Fedor Uporov <fuporov.vstack@gmail.com> Contributions-by: Stuart Maybee <stuart.maybee@comcast.net> Contributions-by: Thorsten Behrens <tbehrens@outlook.com> Contributions-by: Fmstrat <nospam@nowsci.com> Contributions-by: Don Brady <dev.fs.zfs@gmail.com> Signed-off-by: Don Brady <dev.fs.zfs@gmail.com> Closes #15022
177 lines
4.9 KiB
C
177 lines
4.9 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or https://opensource.org/licenses/CDDL-1.0.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
/*
|
|
* Copyright (C) 2016 Gvozden Neskovic <neskovic@compeng.uni-frankfurt.de>.
|
|
*/
|
|
|
|
#ifndef _SYS_VDEV_RAIDZ_H
|
|
#define _SYS_VDEV_RAIDZ_H
|
|
|
|
#include <sys/types.h>
|
|
#include <sys/zfs_rlock.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
struct zio;
|
|
struct raidz_col;
|
|
struct raidz_row;
|
|
struct raidz_map;
|
|
struct vdev_raidz;
|
|
struct uberblock;
|
|
#if !defined(_KERNEL)
|
|
struct kernel_param {};
|
|
#endif
|
|
|
|
/*
|
|
* vdev_raidz interface
|
|
*/
|
|
struct raidz_map *vdev_raidz_map_alloc(struct zio *, uint64_t, uint64_t,
|
|
uint64_t);
|
|
struct raidz_map *vdev_raidz_map_alloc_expanded(struct zio *,
|
|
uint64_t, uint64_t, uint64_t, uint64_t, uint64_t, uint64_t, boolean_t);
|
|
void vdev_raidz_map_free(struct raidz_map *);
|
|
void vdev_raidz_free(struct vdev_raidz *);
|
|
void vdev_raidz_generate_parity_row(struct raidz_map *, struct raidz_row *);
|
|
void vdev_raidz_generate_parity(struct raidz_map *);
|
|
void vdev_raidz_reconstruct(struct raidz_map *, const int *, int);
|
|
void vdev_raidz_child_done(zio_t *);
|
|
void vdev_raidz_io_done(zio_t *);
|
|
void vdev_raidz_checksum_error(zio_t *, struct raidz_col *, abd_t *);
|
|
struct raidz_row *vdev_raidz_row_alloc(int);
|
|
void vdev_raidz_reflow_copy_scratch(spa_t *);
|
|
void raidz_dtl_reassessed(vdev_t *);
|
|
|
|
extern const zio_vsd_ops_t vdev_raidz_vsd_ops;
|
|
|
|
/*
|
|
* vdev_raidz_math interface
|
|
*/
|
|
void vdev_raidz_math_init(void);
|
|
void vdev_raidz_math_fini(void);
|
|
const struct raidz_impl_ops *vdev_raidz_math_get_ops(void);
|
|
int vdev_raidz_math_generate(struct raidz_map *, struct raidz_row *);
|
|
int vdev_raidz_math_reconstruct(struct raidz_map *, struct raidz_row *,
|
|
const int *, const int *, const int);
|
|
int vdev_raidz_impl_set(const char *);
|
|
|
|
typedef struct vdev_raidz_expand {
|
|
uint64_t vre_vdev_id;
|
|
|
|
kmutex_t vre_lock;
|
|
kcondvar_t vre_cv;
|
|
|
|
/*
|
|
* How much i/o is outstanding (issued and not completed).
|
|
*/
|
|
uint64_t vre_outstanding_bytes;
|
|
|
|
/*
|
|
* Next offset to issue i/o for.
|
|
*/
|
|
uint64_t vre_offset;
|
|
|
|
/*
|
|
* Lowest offset of a failed expansion i/o. The expansion will retry
|
|
* from here. Once the expansion thread notices the failure and exits,
|
|
* vre_failed_offset is reset back to UINT64_MAX, and
|
|
* vre_waiting_for_resilver will be set.
|
|
*/
|
|
uint64_t vre_failed_offset;
|
|
boolean_t vre_waiting_for_resilver;
|
|
|
|
/*
|
|
* Offset that is completing each txg
|
|
*/
|
|
uint64_t vre_offset_pertxg[TXG_SIZE];
|
|
|
|
/*
|
|
* Bytes copied in each txg.
|
|
*/
|
|
uint64_t vre_bytes_copied_pertxg[TXG_SIZE];
|
|
|
|
/*
|
|
* The rangelock prevents normal read/write zio's from happening while
|
|
* there are expansion (reflow) i/os in progress to the same offsets.
|
|
*/
|
|
zfs_rangelock_t vre_rangelock;
|
|
|
|
/*
|
|
* These fields are stored on-disk in the vdev_top_zap:
|
|
*/
|
|
dsl_scan_state_t vre_state;
|
|
uint64_t vre_start_time;
|
|
uint64_t vre_end_time;
|
|
uint64_t vre_bytes_copied;
|
|
} vdev_raidz_expand_t;
|
|
|
|
typedef struct vdev_raidz {
|
|
/*
|
|
* Number of child vdevs when this raidz vdev was created (i.e. before
|
|
* any raidz expansions).
|
|
*/
|
|
int vd_original_width;
|
|
|
|
/*
|
|
* The current number of child vdevs, which may be more than the
|
|
* original width if an expansion is in progress or has completed.
|
|
*/
|
|
int vd_physical_width;
|
|
|
|
int vd_nparity;
|
|
|
|
/*
|
|
* Tree of reflow_node_t's. The lock protects the avl tree only.
|
|
* The reflow_node_t's describe completed expansions, and are used
|
|
* to determine the logical width given a block's birth time.
|
|
*/
|
|
avl_tree_t vd_expand_txgs;
|
|
kmutex_t vd_expand_lock;
|
|
|
|
/*
|
|
* If this vdev is being expanded, spa_raidz_expand is set to this
|
|
*/
|
|
vdev_raidz_expand_t vn_vre;
|
|
} vdev_raidz_t;
|
|
|
|
extern int vdev_raidz_attach_check(vdev_t *);
|
|
extern void vdev_raidz_attach_sync(void *, dmu_tx_t *);
|
|
extern void spa_start_raidz_expansion_thread(spa_t *);
|
|
extern int spa_raidz_expand_get_stats(spa_t *, pool_raidz_expand_stat_t *);
|
|
extern int vdev_raidz_load(vdev_t *);
|
|
|
|
/* RAIDZ scratch area pause points (for testing) */
|
|
#define RAIDZ_EXPAND_PAUSE_NONE 0
|
|
#define RAIDZ_EXPAND_PAUSE_PRE_SCRATCH_1 1
|
|
#define RAIDZ_EXPAND_PAUSE_PRE_SCRATCH_2 2
|
|
#define RAIDZ_EXPAND_PAUSE_PRE_SCRATCH_3 3
|
|
#define RAIDZ_EXPAND_PAUSE_SCRATCH_VALID 4
|
|
#define RAIDZ_EXPAND_PAUSE_SCRATCH_REFLOWED 5
|
|
#define RAIDZ_EXPAND_PAUSE_SCRATCH_POST_REFLOW_1 6
|
|
#define RAIDZ_EXPAND_PAUSE_SCRATCH_POST_REFLOW_2 7
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SYS_VDEV_RAIDZ_H */
|