mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-18 02:20:59 +03:00
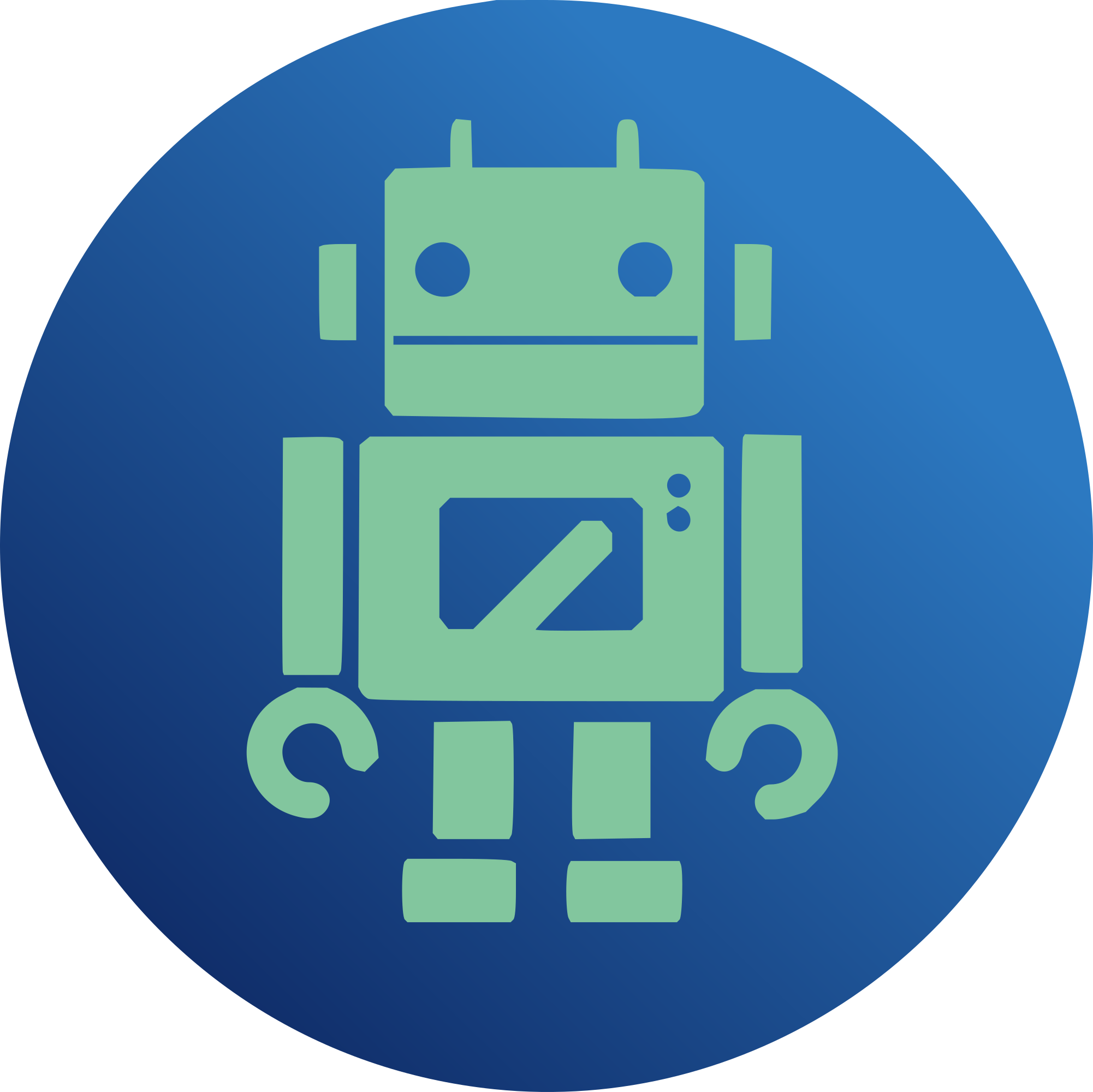
This PR adds two new compression types, based on ZStandard: - zstd: A basic ZStandard compression algorithm Available compression. Levels for zstd are zstd-1 through zstd-19, where the compression increases with every level, but speed decreases. - zstd-fast: A faster version of the ZStandard compression algorithm zstd-fast is basically a "negative" level of zstd. The compression decreases with every level, but speed increases. Available compression levels for zstd-fast: - zstd-fast-1 through zstd-fast-10 - zstd-fast-20 through zstd-fast-100 (in increments of 10) - zstd-fast-500 and zstd-fast-1000 For more information check the man page. Implementation details: Rather than treat each level of zstd as a different algorithm (as was done historically with gzip), the block pointer `enum zio_compress` value is simply zstd for all levels, including zstd-fast, since they all use the same decompression function. The compress= property (a 64bit unsigned integer) uses the lower 7 bits to store the compression algorithm (matching the number of bits used in a block pointer, as the 8th bit was borrowed for embedded block pointers). The upper bits are used to store the compression level. It is necessary to be able to determine what compression level was used when later reading a block back, so the concept used in LZ4, where the first 32bits of the on-disk value are the size of the compressed data (since the allocation is rounded up to the nearest ashift), was extended, and we store the version of ZSTD and the level as well as the compressed size. This value is returned when decompressing a block, so that if the block needs to be recompressed (L2ARC, nop-write, etc), that the same parameters will be used to result in the matching checksum. All of the internal ZFS code ( `arc_buf_hdr_t`, `objset_t`, `zio_prop_t`, etc.) uses the separated _compress and _complevel variables. Only the properties ZAP contains the combined/bit-shifted value. The combined value is split when the compression_changed_cb() callback is called, and sets both objset members (os_compress and os_complevel). The userspace tools all use the combined/bit-shifted value. Additional notes: zdb can now also decode the ZSTD compression header (flag -Z) and inspect the size, version and compression level saved in that header. For each record, if it is ZSTD compressed, the parameters of the decoded compression header get printed. ZSTD is included with all current tests and new tests are added as-needed. Per-dataset feature flags now get activated when the property is set. If a compression algorithm requires a feature flag, zfs activates the feature when the property is set, rather than waiting for the first block to be born. This is currently only used by zstd but can be extended as needed. Portions-Sponsored-By: The FreeBSD Foundation Co-authored-by: Allan Jude <allanjude@freebsd.org> Co-authored-by: Brian Behlendorf <behlendorf1@llnl.gov> Co-authored-by: Sebastian Gottschall <s.gottschall@dd-wrt.com> Co-authored-by: Kjeld Schouten-Lebbing <kjeld@schouten-lebbing.nl> Co-authored-by: Michael Niewöhner <foss@mniewoehner.de> Signed-off-by: Allan Jude <allan@klarasystems.com> Signed-off-by: Allan Jude <allanjude@freebsd.org> Signed-off-by: Brian Behlendorf <behlendorf1@llnl.gov> Signed-off-by: Sebastian Gottschall <s.gottschall@dd-wrt.com> Signed-off-by: Kjeld Schouten-Lebbing <kjeld@schouten-lebbing.nl> Signed-off-by: Michael Niewöhner <foss@mniewoehner.de> Closes #6247 Closes #9024 Closes #10277 Closes #10278
199 lines
5.9 KiB
C
199 lines
5.9 KiB
C
/*
|
|
* CDDL HEADER START
|
|
*
|
|
* The contents of this file are subject to the terms of the
|
|
* Common Development and Distribution License (the "License").
|
|
* You may not use this file except in compliance with the License.
|
|
*
|
|
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
|
|
* or http://www.opensolaris.org/os/licensing.
|
|
* See the License for the specific language governing permissions
|
|
* and limitations under the License.
|
|
*
|
|
* When distributing Covered Code, include this CDDL HEADER in each
|
|
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
|
|
* If applicable, add the following below this CDDL HEADER, with the
|
|
* fields enclosed by brackets "[]" replaced with your own identifying
|
|
* information: Portions Copyright [yyyy] [name of copyright owner]
|
|
*
|
|
* CDDL HEADER END
|
|
*/
|
|
|
|
/*
|
|
* Copyright 2009 Sun Microsystems, Inc. All rights reserved.
|
|
* Copyright (c) 2019, Allan Jude
|
|
* Copyright (c) 2019, Klara Inc.
|
|
* Use is subject to license terms.
|
|
* Copyright (c) 2015, 2016 by Delphix. All rights reserved.
|
|
*/
|
|
|
|
#ifndef _SYS_ZIO_COMPRESS_H
|
|
#define _SYS_ZIO_COMPRESS_H
|
|
|
|
#include <sys/abd.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
enum zio_compress {
|
|
ZIO_COMPRESS_INHERIT = 0,
|
|
ZIO_COMPRESS_ON,
|
|
ZIO_COMPRESS_OFF,
|
|
ZIO_COMPRESS_LZJB,
|
|
ZIO_COMPRESS_EMPTY,
|
|
ZIO_COMPRESS_GZIP_1,
|
|
ZIO_COMPRESS_GZIP_2,
|
|
ZIO_COMPRESS_GZIP_3,
|
|
ZIO_COMPRESS_GZIP_4,
|
|
ZIO_COMPRESS_GZIP_5,
|
|
ZIO_COMPRESS_GZIP_6,
|
|
ZIO_COMPRESS_GZIP_7,
|
|
ZIO_COMPRESS_GZIP_8,
|
|
ZIO_COMPRESS_GZIP_9,
|
|
ZIO_COMPRESS_ZLE,
|
|
ZIO_COMPRESS_LZ4,
|
|
ZIO_COMPRESS_ZSTD,
|
|
ZIO_COMPRESS_FUNCTIONS
|
|
};
|
|
|
|
/* Compression algorithms that have levels */
|
|
#define ZIO_COMPRESS_HASLEVEL(compress) ((compress == ZIO_COMPRESS_ZSTD || \
|
|
(compress >= ZIO_COMPRESS_GZIP_1 && \
|
|
compress <= ZIO_COMPRESS_GZIP_9)))
|
|
|
|
#define ZIO_COMPLEVEL_INHERIT 0
|
|
#define ZIO_COMPLEVEL_DEFAULT 255
|
|
|
|
enum zio_zstd_levels {
|
|
ZIO_ZSTD_LEVEL_INHERIT = 0,
|
|
ZIO_ZSTD_LEVEL_1,
|
|
#define ZIO_ZSTD_LEVEL_MIN ZIO_ZSTD_LEVEL_1
|
|
ZIO_ZSTD_LEVEL_2,
|
|
ZIO_ZSTD_LEVEL_3,
|
|
#define ZIO_ZSTD_LEVEL_DEFAULT ZIO_ZSTD_LEVEL_3
|
|
ZIO_ZSTD_LEVEL_4,
|
|
ZIO_ZSTD_LEVEL_5,
|
|
ZIO_ZSTD_LEVEL_6,
|
|
ZIO_ZSTD_LEVEL_7,
|
|
ZIO_ZSTD_LEVEL_8,
|
|
ZIO_ZSTD_LEVEL_9,
|
|
ZIO_ZSTD_LEVEL_10,
|
|
ZIO_ZSTD_LEVEL_11,
|
|
ZIO_ZSTD_LEVEL_12,
|
|
ZIO_ZSTD_LEVEL_13,
|
|
ZIO_ZSTD_LEVEL_14,
|
|
ZIO_ZSTD_LEVEL_15,
|
|
ZIO_ZSTD_LEVEL_16,
|
|
ZIO_ZSTD_LEVEL_17,
|
|
ZIO_ZSTD_LEVEL_18,
|
|
ZIO_ZSTD_LEVEL_19,
|
|
#define ZIO_ZSTD_LEVEL_MAX ZIO_ZSTD_LEVEL_19
|
|
ZIO_ZSTD_LEVEL_RESERVE = 101, /* Leave room for new positive levels */
|
|
ZIO_ZSTD_LEVEL_FAST, /* Fast levels are negative */
|
|
ZIO_ZSTD_LEVEL_FAST_1,
|
|
#define ZIO_ZSTD_LEVEL_FAST_DEFAULT ZIO_ZSTD_LEVEL_FAST_1
|
|
ZIO_ZSTD_LEVEL_FAST_2,
|
|
ZIO_ZSTD_LEVEL_FAST_3,
|
|
ZIO_ZSTD_LEVEL_FAST_4,
|
|
ZIO_ZSTD_LEVEL_FAST_5,
|
|
ZIO_ZSTD_LEVEL_FAST_6,
|
|
ZIO_ZSTD_LEVEL_FAST_7,
|
|
ZIO_ZSTD_LEVEL_FAST_8,
|
|
ZIO_ZSTD_LEVEL_FAST_9,
|
|
ZIO_ZSTD_LEVEL_FAST_10,
|
|
ZIO_ZSTD_LEVEL_FAST_20,
|
|
ZIO_ZSTD_LEVEL_FAST_30,
|
|
ZIO_ZSTD_LEVEL_FAST_40,
|
|
ZIO_ZSTD_LEVEL_FAST_50,
|
|
ZIO_ZSTD_LEVEL_FAST_60,
|
|
ZIO_ZSTD_LEVEL_FAST_70,
|
|
ZIO_ZSTD_LEVEL_FAST_80,
|
|
ZIO_ZSTD_LEVEL_FAST_90,
|
|
ZIO_ZSTD_LEVEL_FAST_100,
|
|
ZIO_ZSTD_LEVEL_FAST_500,
|
|
ZIO_ZSTD_LEVEL_FAST_1000,
|
|
#define ZIO_ZSTD_LEVEL_FAST_MAX ZIO_ZSTD_LEVEL_FAST_1000
|
|
ZIO_ZSTD_LEVEL_AUTO = 251, /* Reserved for future use */
|
|
ZIO_ZSTD_LEVEL_LEVELS
|
|
};
|
|
|
|
/* Forward Declaration to avoid visibility problems */
|
|
struct zio_prop;
|
|
|
|
/* Common signature for all zio compress functions. */
|
|
typedef size_t zio_compress_func_t(void *src, void *dst,
|
|
size_t s_len, size_t d_len, int);
|
|
/* Common signature for all zio decompress functions. */
|
|
typedef int zio_decompress_func_t(void *src, void *dst,
|
|
size_t s_len, size_t d_len, int);
|
|
/* Common signature for all zio decompress and get level functions. */
|
|
typedef int zio_decompresslevel_func_t(void *src, void *dst,
|
|
size_t s_len, size_t d_len, uint8_t *level);
|
|
/* Common signature for all zio get-compression-level functions. */
|
|
typedef int zio_getlevel_func_t(void *src, size_t s_len, uint8_t *level);
|
|
|
|
|
|
/*
|
|
* Common signature for all zio decompress functions using an ABD as input.
|
|
* This is helpful if you have both compressed ARC and scatter ABDs enabled,
|
|
* but is not a requirement for all compression algorithms.
|
|
*/
|
|
typedef int zio_decompress_abd_func_t(abd_t *src, void *dst,
|
|
size_t s_len, size_t d_len, int);
|
|
/*
|
|
* Information about each compression function.
|
|
*/
|
|
typedef const struct zio_compress_info {
|
|
char *ci_name;
|
|
int ci_level;
|
|
zio_compress_func_t *ci_compress;
|
|
zio_decompress_func_t *ci_decompress;
|
|
zio_decompresslevel_func_t *ci_decompress_level;
|
|
} zio_compress_info_t;
|
|
|
|
extern zio_compress_info_t zio_compress_table[ZIO_COMPRESS_FUNCTIONS];
|
|
|
|
/*
|
|
* lz4 compression init & free
|
|
*/
|
|
extern void lz4_init(void);
|
|
extern void lz4_fini(void);
|
|
|
|
/*
|
|
* Compression routines.
|
|
*/
|
|
extern size_t lzjb_compress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern int lzjb_decompress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern size_t gzip_compress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern int gzip_decompress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern size_t zle_compress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern int zle_decompress(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern size_t lz4_compress_zfs(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
extern int lz4_decompress_zfs(void *src, void *dst, size_t s_len, size_t d_len,
|
|
int level);
|
|
|
|
/*
|
|
* Compress and decompress data if necessary.
|
|
*/
|
|
extern size_t zio_compress_data(enum zio_compress c, abd_t *src, void *dst,
|
|
size_t s_len, uint8_t level);
|
|
extern int zio_decompress_data(enum zio_compress c, abd_t *src, void *dst,
|
|
size_t s_len, size_t d_len, uint8_t *level);
|
|
extern int zio_decompress_data_buf(enum zio_compress c, void *src, void *dst,
|
|
size_t s_len, size_t d_len, uint8_t *level);
|
|
extern int zio_compress_to_feature(enum zio_compress comp);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SYS_ZIO_COMPRESS_H */
|