mirror of
https://git.proxmox.com/git/mirror_zfs.git
synced 2024-11-17 01:51:00 +03:00
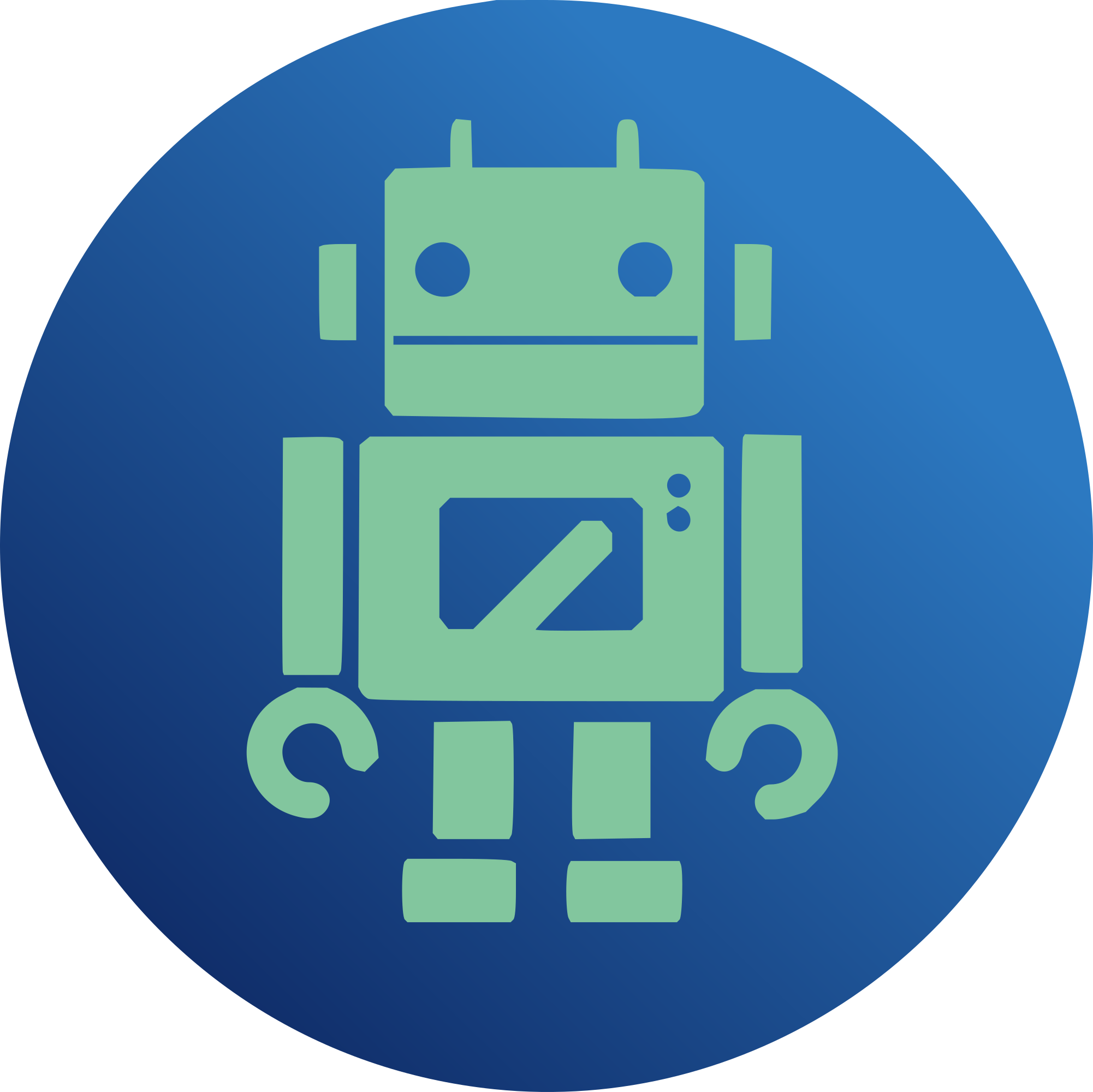
git-svn-id: https://outreach.scidac.gov/svn/spl/trunk@17 7e1ea52c-4ff2-0310-8f11-9dd32ca42a1c
64 lines
1.3 KiB
C
64 lines
1.3 KiB
C
#ifndef _SPL_TIME_H
|
|
#define _SPL_TIME_H
|
|
|
|
/*
|
|
* Structure returned by gettimeofday(2) system call,
|
|
* and used in other calls.
|
|
*/
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/time.h>
|
|
#include "spl-types.h"
|
|
|
|
extern unsigned long long monotonic_clock(void);
|
|
typedef struct timespec timestruc_t; /* definition per SVr4 */
|
|
typedef longlong_t hrtime_t;
|
|
|
|
#define TIME32_MAX INT32_MAX
|
|
#define TIME32_MIN INT32_MIN
|
|
|
|
#define SEC 1
|
|
#define MILLISEC 1000
|
|
#define MICROSEC 1000000
|
|
#define NANOSEC 1000000000
|
|
|
|
#define hz \
|
|
({ \
|
|
BUG_ON(HZ < 100 || HZ > MICROSEC); \
|
|
HZ; \
|
|
})
|
|
|
|
#define gethrestime(ts) getnstimeofday((ts))
|
|
|
|
static __inline__ hrtime_t
|
|
gethrtime(void) {
|
|
/* BUG_ON(cur_timer == timer_none); */
|
|
|
|
/* Solaris expects a long long here but monotonic_clock() returns an
|
|
* unsigned long long. Note that monotonic_clock() returns the number
|
|
* of nanoseconds passed since kernel initialization. Even for a signed
|
|
* long long this will not "go negative" for ~292 years.
|
|
*/
|
|
return monotonic_clock();
|
|
}
|
|
|
|
static __inline__ time_t
|
|
gethrestime_sec(void)
|
|
{
|
|
timestruc_t now;
|
|
|
|
gethrestime(&now);
|
|
return (now.tv_sec);
|
|
}
|
|
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SPL_TIME_H */
|